#include<stdio.h> #include<stdlib.h> // 定义二叉排序树的结构体 typedef struct BSTNode { int key; struct BSTNode* left; struct BSTNode* right; }BSTNode; // 插入节点 BSTNode* insert(BSTNode* root, int key) { if (root == NULL) { root = (BSTNode*)malloc(sizeof(BSTNode)); root->key = key; root->left = root->right = NULL; } else if (key < root->key) { root->left = insert(root->left, key); } else if (key > root->key) { root->right = insert(root->right, key); } return root; } // 中序遍历 void inorder(BSTNode* root) { if (root != NULL) { inorder(root->left); printf("%d ", root->key); inorder(root->right); } } int main() { int keys[] = { 16,5,17,29,11,3,15,20 }; BSTNode* root = NULL; int n = sizeof(keys) / sizeof(int); for (int i = 0; i < n; i++) { root = insert(root, keys[i]); } inorder(root); return 0; }根据代码画出完整的文字版流程图
时间: 2023-12-27 13:03:32 浏览: 50
开始
定义二叉排序树结构体
定义BSTNode结构体,包含键值key和左右子节点left和right
插入节点
定义insert函数,传入根节点root和键值key
如果根节点为空,则新建一个节点作为根节点,键值为key,左右子节点为空
如果键值小于根节点的键值,则将其插入根节点的左子树中,递归调用insert函数
如果键值大于根节点的键值,则将其插入根节点的右子树中,递归调用insert函数
返回根节点
中序遍历
定义inorder函数,传入根节点root
如果根节点不为空,则:
先递归遍历左子树
输出根节点的键值
再递归遍历右子树
主函数
定义keys数组,包含要插入的键值
定义根节点root为空
计算keys数组的大小n
对于keys数组中的每个元素,调用insert函数,将其插入二叉排序树中
调用inorder函数,进行中序遍历,输出结果
结束
相关问题
#include <stdio.h>#include <stdlib.h>typedef int TElemType;typedef int Status;#define OK 1#define ERROR -1typedef struct BSTNode{ TElemType data; struct BSTNode *leftchild,*rightchild;}BSTNode,*BSTree;Status CreateBST(BSTree &T,int x) { if(T==NULL) { T = (BSTree)malloc(sizeof(BSTNode)); T->data=x; T->leftchild=T->rightchild=NULL; return OK; } if (x < T->data) { CreateBST(T->leftchild, x); } else { CreateBST(T->rightchild, x); } return OK;}Status print(BSTree T) { if(T) { print(T->leftchild); printf("%d ",T->data); print(T->rightchild); } return OK;}int main() { int a[100]; int n,i; BSTree T=NULL; scanf("%d",&n); for(i=0;i<n;i++) { scanf("%d",&a[i]); } for(i=0;i<n;i++) { CreateBST(T,a[i]); } print(T); printf("\n"); return 0;}
这是一段使用C语言实现的二叉搜索树的创建与遍历代码。在该代码中,定义了二叉搜索树节点的结构体BSTNode,包含数据域和左右孩子指针。使用CreateBST函数来创建二叉搜索树,print函数来进行中序遍历输出二叉搜索树的节点值。在main函数中,先输入节点个数n和n个节点的值,然后通过CreateBST函数创建二叉搜索树,并用print函数输出二叉搜索树的节点值。
1 实验目的 (1)掌握查找的不同方法,并能用高级语言实现查找算法; (2)熟练掌握二叉排序树的构造和查找方法; 2 实验内容 设计一个算法读入一串整数,然后构造二叉排序树,进行查找。 3 实验步骤 (1)从空的二叉树开始,每输入一个节点数据,就建立一个新节点插入到当前已生成的二叉排序树中; (2)在二叉排序树中查找某一结点; (3)删除任一指定的结点 4 类型定义 typedef struct Node /*二叉排序树节点结构*/ { KeyType key ; /*关键字值*/ struct node *lchild, *rchild; /*左右指针*/ }BSTNode;
下面是一个关于实现算法读入一串整数,构造二叉排序树,并进行查找和删除的C语言程序,其中包含了给出的节点结构体类型定义:
```
#include <stdio.h>
#include <stdlib.h>
#define TRUE 1
#define FALSE 0
typedef int KeyType;
typedef struct Node {
KeyType key;
struct Node *lchild, *rchild;
} BSTNode;
BSTNode *createBST() {
return NULL;
}
BSTNode *insertBST(BSTNode *root, KeyType key) {
if (root == NULL) {
BSTNode *newNode = (BSTNode *)malloc(sizeof(BSTNode));
newNode->key = key;
newNode->lchild = newNode->rchild = NULL;
return newNode;
} else if (key < root->key) {
root->lchild = insertBST(root->lchild, key);
} else if (key > root->key) {
root->rchild = insertBST(root->rchild, key);
}
return root;
}
BSTNode *searchBST(BSTNode *root, KeyType key) {
if (root == NULL || root->key == key) {
return root;
} else if (key < root->key) {
return searchBST(root->lchild, key);
} else {
return searchBST(root->rchild, key);
}
}
BSTNode *findMin(BSTNode *root) {
if (root == NULL) {
return NULL;
} else if (root->lchild == NULL) {
return root;
} else {
return findMin(root->lchild);
}
}
BSTNode *deleteBST(BSTNode *root, KeyType key) {
if (root == NULL) {
return NULL;
} else if (key < root->key) {
root->lchild = deleteBST(root->lchild, key);
} else if (key > root->key) {
root->rchild = deleteBST(root->rchild, key);
} else if (root->lchild && root->rchild) {
BSTNode *tmp = findMin(root->rchild);
root->key = tmp->key;
root->rchild = deleteBST(root->rchild, root->key);
} else {
BSTNode *tmp = root;
if (root->lchild == NULL) {
root = root->rchild;
} else if (root->rchild == NULL) {
root = root->lchild;
}
free(tmp);
}
return root;
}
void preOrder(BSTNode *root) {
if (root != NULL) {
printf("%d ", root->key);
preOrder(root->lchild);
preOrder(root->rchild);
}
}
void inOrder(BSTNode *root) {
if (root != NULL) {
inOrder(root->lchild);
printf("%d ", root->key);
inOrder(root->rchild);
}
}
void postOrder(BSTNode *root) {
if (root != NULL) {
postOrder(root->lchild);
postOrder(root->rchild);
printf("%d ", root->key);
}
}
int main() {
BSTNode *root = createBST();
int n, i;
KeyType key;
printf("请输入数字个数:");
scanf("%d", &n);
printf("请输入数字:");
for (i = 0; i < n; i++) {
scanf("%d", &key);
root = insertBST(root, key);
}
printf("前序遍历:");
preOrder(root);
printf("\n");
printf("中序遍历:");
inOrder(root);
printf("\n");
printf("后序遍历:");
postOrder(root);
printf("\n");
printf("请输入要查找的数字:");
scanf("%d", &key);
BSTNode *p = searchBST(root, key);
if (p != NULL) {
printf("查找成功,数字%d存在于树中!\n", p->key);
} else {
printf("查找失败,数字%d不存在于树中!\n", key);
}
printf("请输入要删除的数字:");
scanf("%d", &key);
root = deleteBST(root, key);
printf("删除后的中序遍历:");
inOrder(root);
printf("\n");
return 0;
}
```
在这个程序中,我们首先定义了二叉排序树节点的结构体类型,包括关键字值和左右指针。然后,我们实现了创建二叉排序树、插入节点、查找节点、删除节点等操作。最后,我们采用前序、中序、后序遍历的方式输出二叉排序树中所有节点的关键字值,并给出了查找和删除的示例。
希望这个程序能够帮助您理解二叉排序树的构造和操作,如有任何问题和疑问,欢迎继续追问。
阅读全文
相关推荐
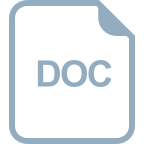
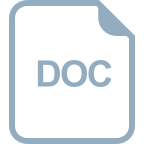
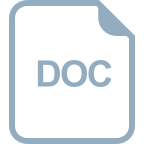














