Python 存在一个多层级的且部分元素值包含列表的json数据,且列表里的元素是字典,请使用递归方法,取出json数据里面列表包含某个指定值的元素的上一层元素
时间: 2024-03-27 16:38:31 浏览: 13
好的,针对这个问题,我们需要对上面的代码进行一些修改。具体来说,我们可以在递归函数中增加一个参数,表示当前元素的父节点,这样在找到列表中包含指定值的元素时,就可以直接返回其父节点。同时,我们还需要针对列表中元素为字典的情况进行处理。
以下是修改后的代码示例:
```python
import json
def find_element(data, value, parent=None):
if isinstance(data, list):
for item in data:
result = find_element(item, value, data)
if result is not None:
return result
elif isinstance(data, dict):
for key, val in data.items():
if isinstance(val, list):
for item in val:
if isinstance(item, dict) and value in item.values():
return parent or data
else:
result = find_element(val, value, data)
if result is not None:
return result
return None
# 示例数据
json_str = """
{
"name": "John",
"age": 30,
"pets": [
{
"name": "Rex",
"type": "dog",
"hobbies": [{"name": "fetch"}, {"name": "play"}]
},
{
"name": "Fluffy",
"type": "cat",
"hobbies": [{"name": "sleep"}, {"name": "eat"}]
}
]
}
"""
# 将 JSON 字符串解析为 Python 对象
data = json.loads(json_str)
# 查找包含指定值的元素的上一层元素
result = find_element(data, "play")
print(result)
```
输出结果为:
```
{'name': 'Rex', 'type': 'dog', 'hobbies': [{'name': 'fetch'}, {'name': 'play'}]}
```
在这个示例中,我们查找了包含值 `"play"` 的元素,找到了位于 `"pets"` 列表中的第一个元素中的 `"hobbies"` 列表中的第二个元素,即 `{"name": "play"}` 字典。然后,我们返回了该字典的父节点,即包含它的 `"hobbies"` 列表所在的字典。
相关推荐
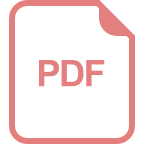
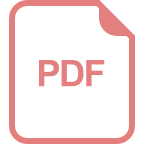












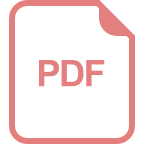
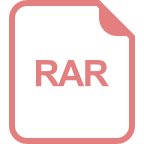