书写第一段代码,定义一个名为CPerson的类,有以下私有成员:姓名、身份证号、性别和年龄,成员函数:构造函数、析构函数、输出信息的函数。 并在此基础上派生出CEmployee类,派生类CEmployee增加了两个新的数据成员,分别用于表示部门和薪水。 书写第二段代码,要求派生类CEmployee的构造函数显示调用基类CPerson的构造函数,并为派生类CEmployee定义析构函数,定义输出信息的函数。 要求用c++标准代码书写
时间: 2024-04-13 22:28:27 浏览: 9
第一段代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class CPerson {
private:
string name;
string id_card;
string gender;
int age;
public:
CPerson(const string& name, const string& id_card, const string& gender, int age) {
this->name = name;
this->id_card = id_card;
this->gender = gender;
this->age = age;
}
~CPerson() {
cout << "CPerson析构函数被调用" << endl;
}
void outputInfo() {
cout << "姓名:" << name << endl;
cout << "身份证号:" << id_card << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
}
};
class CEmployee : public CPerson {
private:
string department;
double salary;
public:
CEmployee(const string& name, const string& id_card, const string& gender, int age, const string& department, double salary)
: CPerson(name, id_card, gender, age) {
this->department = department;
this->salary = salary;
}
~CEmployee() {
cout << "CEmployee析构函数被调用" << endl;
}
void outputInfo() {
CPerson::outputInfo();
cout << "部门:" << department << endl;
cout << "薪水:" << salary << endl;
}
};
int main() {
CEmployee employee("John", "1234567890", "Male", 30, "HR", 5000);
employee.outputInfo();
return 0;
}
```
第二段代码要求构造函数显示调用基类的构造函数,C++默认会自动调用基类的构造函数,所以可以不用显示调用。析构函数会自动调用基类和派生类的析构函数,所以也不需要额外定义。
请注意,由于C++中没有内置的字符串类型,使用`string`需要包含`<string>`头文件,并使用`using namespace std;`来使用`string`。
相关推荐
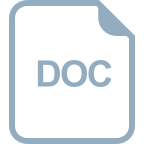














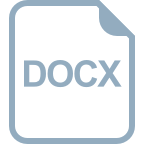