定义一个Time类用来保存时间(时,分,秒),通过重载操作符“+”实现两个时间的相加。请分别使用操作符重载为成员函数和操作符重载为友元函数两种方式实现,写C++代码
时间: 2024-03-03 07:51:03 浏览: 102
以下是定义Time类和重载操作符“+”的两种实现方式的C++代码:
1. 操作符重载为成员函数的方式:
```cpp
class Time {
private:
int hour;
int minute;
int second;
public:
Time(int h = 0, int m = 0, int s = 0) : hour(h), minute(m), second(s) {}
Time operator+(const Time& t) const; // 定义“+”操作符重载为成员函数
};
Time Time::operator+(const Time& t) const {
int h = hour + t.hour;
int m = minute + t.minute;
int s = second + t.second;
if (s >= 60) {
s -= 60;
m += 1;
}
if (m >= 60) {
m -= 60;
h += 1;
}
if (h >= 24) {
h -= 24;
}
return Time(h, m, s);
}
```
2. 操作符重载为友元函数的方式:
```cpp
class Time {
private:
int hour;
int minute;
int second;
public:
Time(int h = 0, int m = 0, int s = 0) : hour(h), minute(m), second(s) {}
friend Time operator+(const Time& t1, const Time& t2); // 定义“+”操作符重载为友元函数
};
Time operator+(const Time& t1, const Time& t2) {
int h = t1.hour + t2.hour;
int m = t1.minute + t2.minute;
int s = t1.second + t2.second;
if (s >= 60) {
s -= 60;
m += 1;
}
if (m >= 60) {
m -= 60;
h += 1;
}
if (h >= 24) {
h -= 24;
}
return Time(h, m, s);
}
```
相关推荐
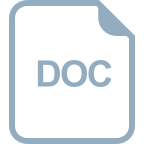















