c++定义一个Time类用来保存时间(时,分,秒),通过重载操作符“+”实现两个时间的相加。
时间: 2024-12-25 12:36:11 浏览: 22
在 C++ 中,你可以定义一个 `Time` 类来表示时间,并使用运算符重载功能来实现两个时间的相加。首先,你需要包含必要的头文件,然后定义类、成员变量(如小时、分钟和秒),以及构造函数、赋值运算符等。这里是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
class Time {
private:
int hours;
int minutes;
int seconds;
public:
// 构造函数,设置默认时间为0
Time() : hours(0), minutes(0), seconds(0) {}
// 参数初始化构造函数
Time(int h, int m, int s) : hours(h), minutes(m), seconds(s) {}
// 赋值运算符,用于复制时间
Time& operator=(const Time& other) {
if (this != &other) {
hours = other.hours;
minutes = other.minutes;
seconds = other.seconds;
}
return *this;
}
// 运算符重载 '+'
Time operator+(const Time& other) const {
int totalSeconds = hours * 3600 + minutes * 60 + seconds + other.hours * 3600 + other.minutes * 60 + other.seconds;
int totalHours = totalSeconds / 3600;
int remainingSeconds = totalSeconds % 3600;
int totalMinutes = remainingSeconds / 60;
int finalSeconds = remainingSeconds % 60;
Time result(totalHours, totalMinutes, finalSeconds);
return result;
}
// 重载 << 运算符便于打印
friend ostream& operator<<(ostream& os, const Time& time) {
os << time.hours << "小时 " << time.minutes << "分钟 " << time.seconds << "秒";
return os;
}
};
int main() {
Time t1(10, 30, 45);
Time t2(2, 15, 30);
Time sum = t1 + t2; // 相加
cout << "t1 和 t2 的和: " << sum << endl;
return 0;
}
```
在这个例子中,我们定义了加法 `operator+` 来将两个 `Time` 对象转换成总秒数,然后计算新的小时、分钟和秒数。此外,我们还重载了 `<<` 操作符以便于输出时间和进行调试。
阅读全文
相关推荐




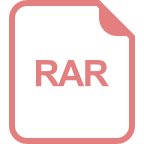












