c++编写一个重载函数实现两个复数相加功能
时间: 2024-12-18 17:31:22 浏览: 4
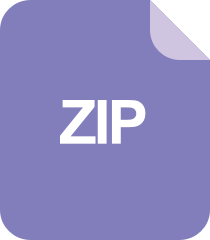
实例003——操作符重载实现编译多态——复数的加法运算.zip
在 C++ 中,我们可以创建一个重载的加法运算符 `+` 或者一个专门的成员函数来实现复数的相加。因为 C++ 不支持直接的运算符重载,所以我们需要定义一个名为 `operator+` 的函数来模拟加法操作。首先,我们需要定义一个复数类(Complex),然后实现它的构造函数、赋值操作符和加法操作。
```cpp
class Complex {
private:
double real;
double imag;
public:
// 构造函数,初始化复数的实部和虚部
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 赋值操作符,让实例化后的复数等于另一个复数
Complex& operator=(const Complex& other) {
if (this != &other) {
real = other.real;
imag = other.imag;
}
return *this;
}
// 加法重载,两个复数相加
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 输出复数的形式,方便查看
friend ostream& operator<<(ostream& os, const Complex& complex) {
return os << complex.real << "+" << complex.imag << "i";
}
};
int main() {
Complex c1(2, 3);
Complex c2(4, -1);
Complex sum = c1 + c2; // 相加操作
cout << "Sum of complex numbers: " << sum << endl;
return 0;
}
```
在这个例子中,我们定义了一个 `Complex` 类,它有 `real` 和 `imag` 成员变量表示实部和虚部。`operator+` 函数实现了两个复数的相加。同时,为了方便输出复数,我们也定义了 `<<` 运算符的重载,使得 `cout` 可以正确显示复数。
阅读全文
相关推荐
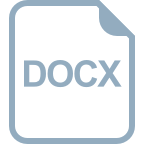
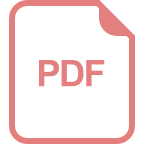
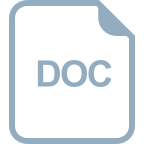




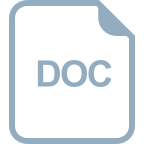
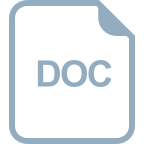
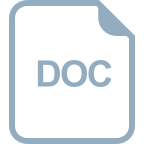
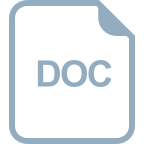






