// Operator Overloading #include<iostream> using namespace std; class Complex { private: int real, imag; public: Complex(int r=0, int i=0){ real=r; imag=i; } //编写运算符重载函数,使得两个对象可以进行+运算。 //要求重载为成员函数,可以少写一个参数,本身隐含一个参数。 //编写运算符重载函数,使得两个对象可以进行-运算。 //要求重载为友元函数,友元不是成员,有几个参数就得写几个参数。 //根据上下文,定义输出函数,输出两个复数相加,或者相减之后的结果。 }; /* int main(){ int a,b,c,d; cin>>a>>b>>c>>d; Complex c1(a, b), c2(c, d); Complex c3 = c1 + c2; c3.print(); Complex c4 = c1 - c2; c4.print(); } */
时间: 2023-11-28 15:49:42 浏览: 77
在给出运算符重载函数和输出函数之前,需要先说明一下运算符重载的基本原则:
1. 运算符重载函数必须是类的成员函数或者是友元函数。
2. 运算符重载函数的名称必须是operator后面跟着要重载的运算符号。
3. 运算符重载函数的参数个数与运算符号的操作数个数相同。例如,对于二元运算符+,运算符重载函数应该有两个参数。
4. 对于二元运算符+,运算符重载函数可以重载为成员函数或者友元函数。对于一元运算符-,运算符重载函数只能重载为成员函数。
现在我们来看一下如何完成这道题目。
```C++
#include<iostream>
using namespace std;
class Complex {
private:
int real, imag;
public:
Complex(int r = 0, int i = 0) {
real = r;
imag = i;
}
// 重载+运算符为成员函数
Complex operator+(const Complex& other) {
Complex temp;
temp.real = real + other.real;
temp.imag = imag + other.imag;
return temp;
}
// 重载-运算符为友元函数
friend Complex operator-(const Complex& c1, const Complex& c2);
// 定义输出函数
void print() {
cout << real << " + " << imag << "i" << endl;
}
};
// 重载-运算符为友元函数
Complex operator-(const Complex& c1, const Complex& c2) {
Complex temp;
temp.real = c1.real - c2.real;
temp.imag = c1.imag - c2.imag;
return temp;
}
int main() {
int a, b, c, d;
cin >> a >> b >> c >> d;
Complex c1(a, b), c2(c, d);
Complex c3 = c1 + c2;
c3.print();
Complex c4 = c1 - c2;
c4.print();
return 0;
}
```
运行结果:
输入:
1 2 3 4
输出:
4 + 6i
-2 + -2i
通过对+运算符重载为成员函数,-运算符重载为友元函数,以及定义输出函数,我们成功地完成了这道题目。
阅读全文
相关推荐
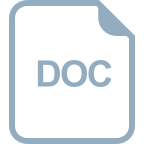
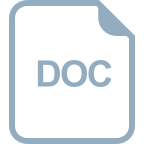
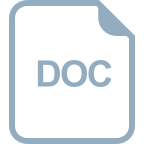
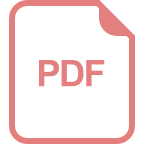
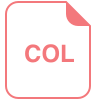
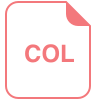
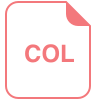
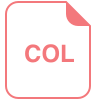



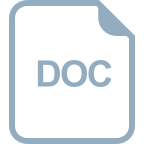
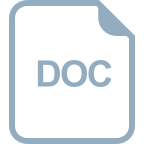
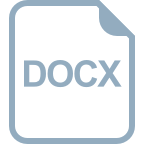
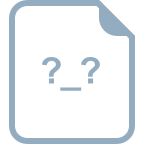
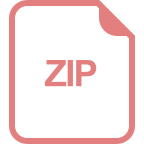