用Python写个人脸识别
时间: 2023-07-28 17:07:47 浏览: 61
好的,我可以为您提供一个简单的人脸识别的Python代码。首先,您需要安装OpenCV和face_recognition库,可以使用以下命令进行安装:
```
pip install opencv-python
pip install face_recognition
```
然后,您可以使用以下代码进行人脸识别:
```python
import cv2
import face_recognition
# 读取已知人脸图像和名称
known_image = face_recognition.load_image_file("known.jpg")
known_encoding = face_recognition.face_encodings(known_image)[0]
known_name = "John"
# 初始化摄像头
video_capture = cv2.VideoCapture(0)
while True:
# 读取摄像头中的图像
ret, frame = video_capture.read()
# 将图像进行缩放,减少运算量
small_frame = cv2.resize(frame, (0, 0), fx=0.25, fy=0.25)
# 将图像从BGR格式转换为RGB格式
rgb_small_frame = small_frame[:, :, ::-1]
# 检测图像中的人脸
face_locations = face_recognition.face_locations(rgb_small_frame)
face_encodings = face_recognition.face_encodings(rgb_small_frame, face_locations)
# 遍历检测到的人脸
for face_encoding in face_encodings:
# 将检测到的人脸与已知人脸进行比较
matches = face_recognition.compare_faces([known_encoding], face_encoding)
# 如果匹配,则显示该人的名字
if matches[0]:
name = known_name
else:
name = "Unknown"
# 在图像中标出人脸和名字
top, right, bottom, left = face_locations[0]
top *= 4
right *= 4
bottom *= 4
left *= 4
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
cv2.rectangle(frame, (left, bottom - 35), (right, bottom), (0, 0, 255), cv2.FILLED)
cv2.putText(frame, name, (left + 6, bottom - 6), cv2.FONT_HERSHEY_SIMPLEX, 1.0, (255, 255, 255), 1)
# 显示图像
cv2.imshow('Video', frame)
# 按下 q 键退出程序
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头和窗口
video_capture.release()
cv2.destroyAllWindows()
```
在该代码中,我们首先读取已知人脸的图像和名称,并对其进行编码。然后,我们初始化摄像头,遍历检测到的人脸,并将其与已知人脸进行比较,最后在图像中标出人脸和名字。您可以根据自己的需要进行修改和调整。
相关推荐













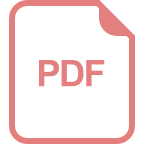
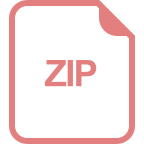