pyqt实现astar算法
时间: 2024-10-01 19:11:42 浏览: 30
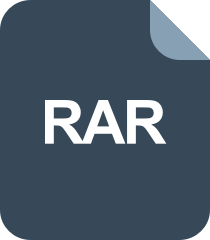
基于PyQt的图像算法开发软件
PyQt是一种Python绑定的跨平台图形用户界面应用程序开发工具集,它结合了Python语言的简洁性和Qt库的强大功能。A*算法(A* Search Algorithm)是一种用于路径finding(寻路)的问题求解算法,特别适合于寻找两点之间的最短路径。
在PyQt中实现A*算法通常会分为以下几个步骤:
1. **数据结构**:创建地图表示,可以使用QGraphicsScene、QGraphicsPolygonItem等Qt组件,将地图格化成节点和边。
2. **类设计**:定义Agent类作为搜索者,包含状态(位置)、目标位置以及开放列表和关闭列表的数据结构。
3. **启发式函数**:编写计算从当前节点到目标节点的估计距离(如曼哈顿距离或欧几里得距离)的函数,这是A*算法的关键部分。
4. **搜索函数**:在A*类中实现搜索过程,包括添加起始节点到开放列表,不断选择当前节点(F值最小),移动并更新节点状态,直到找到终点或开放列表为空。
5. **UI交互**:创建GUI界面,允许用户输入起点和终点,并实时显示搜索结果。
```python
class AStar(QThread):
def __init__(self, start, end, scene):
super().__init__()
self.start = start
self.end = end
self.scene = scene
# ... 其他搜索算法实现 ...
@pyqtSlot()
def run(self):
path = self.search()
for node in path:
# 在场景上绘制节点和路径
self.scene.removeItem(node)
new_node = QGraphicsPolygonItem(path[node], self.scene)
new_node.setPen(Qt.green)
# 使用示例
start_pos = (0, 0)
end_pos = (10, 10)
astar_thread = AStar(start_pos, end_pos, scene)
astar_thread.start()
```
阅读全文
相关推荐
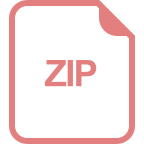
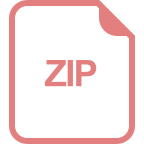
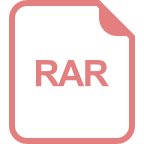

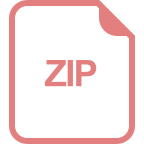
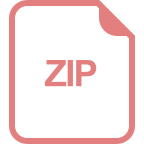
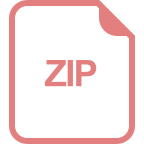
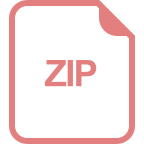
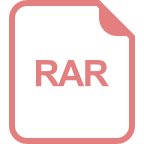
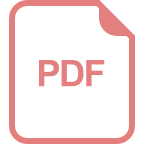
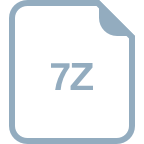
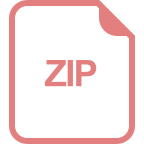
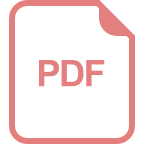
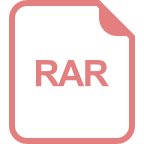
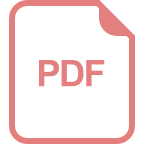
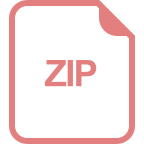