java实现docx文档模板对表格数据填充
时间: 2023-06-24 14:08:20 浏览: 125
使用 Java 实现 Docx 文档模板对表格数据填充的步骤如下:
1. 首先,需要准备好一个 Docx 文档模板,其中包含需要填充数据的表格。
2. 使用 Apache POI 库打开 Docx 文档模板,并读取需要填充数据的表格。
3. 将需要填充的数据保存在 Java 对象中,例如 List<Map<String, String>>,其中每个 Map 对象表示一行表格数据,Map 的 key 表示表格列名,value 表示对应列的数据。
4. 遍历需要填充的数据,逐行对表格进行填充。
5. 在填充表格数据时,需要注意表格中可能存在合并单元格的情况,需要根据合并单元格的信息将数据正确地填充到表格中。
6. 填充完数据后,将修改后的文档保存到磁盘中。
下面是一个简单的示例代码,演示了如何使用 Java 实现 Docx 文档模板对表格数据填充:
```
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.List;
import java.util.Map;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFTable;
import org.apache.poi.xwpf.usermodel.XWPFTableRow;
import org.apache.poi.xwpf.usermodel.XWPFTableCell;
public class DocxTemplateFiller {
public static void fillTableData(String templatePath, String outputPath, List<Map<String, String>> data) throws Exception {
// 打开文档模板
XWPFDocument doc = new XWPFDocument(new FileInputStream(templatePath));
// 获取需要填充数据的表格
XWPFTable table = doc.getTables().get(0);
// 遍历数据,逐行填充表格
for (Map<String, String> row : data) {
XWPFTableRow tableRow = table.createRow();
int colIndex = 0;
for (Map.Entry<String, String> entry : row.entrySet()) {
// 根据列名获取列索引
int rowIndex = getRowIndex(table, 0, entry.getKey(), colIndex);
XWPFTableCell cell = tableRow.getCell(rowIndex);
// 填充单元格数据
cell.setText(entry.getValue());
// 处理合并单元格
int[] mergeRange = getMergeRange(table, rowIndex, table.getNumberOfRows() - 1);
if (mergeRange != null) {
mergeCellsVertically(table, rowIndex, mergeRange[1], mergeRange[0]);
colIndex = mergeRange[1] + 1;
} else {
colIndex = rowIndex + 1;
}
}
}
// 保存修改后的文档
doc.write(new FileOutputStream(outputPath));
doc.close();
}
private static int getRowIndex(XWPFTable table, int rowStartIndex, String colName, int colStartIndex) {
for (int rowIndex = rowStartIndex; rowIndex < table.getNumberOfRows(); rowIndex++) {
XWPFTableRow row = table.getRow(rowIndex);
for (int colIndex = colStartIndex; colIndex < row.getTableCells().size(); colIndex++) {
String cellText = row.getCell(colIndex).getText();
if (cellText.contains(colName)) {
return colIndex;
}
}
}
return -1;
}
private static int[] getMergeRange(XWPFTable table, int rowIndex, int rowEndIndex) {
int[] range = null;
for (int i = rowIndex; i <= rowEndIndex; i++) {
XWPFTableRow row = table.getRow(i);
XWPFTableCell cell = row.getCell(rowIndex);
if (cell != null && cell.getCTTc().isSetTcPr()) {
if (cell.getCTTc().getTcPr().isSetVMerge()) {
int vMergeVal = cell.getCTTc().getTcPr().getVMerge().getVal();
if (vMergeVal == 0) {
range = new int[]{rowIndex, i};
break;
}
}
}
}
return range;
}
private static void mergeCellsVertically(XWPFTable table, int colIndex, int fromRow, int toRow) {
for (int rowIndex = fromRow; rowIndex <= toRow; rowIndex++) {
XWPFTableRow row = table.getRow(rowIndex);
XWPFTableCell cell = row.getCell(colIndex);
if (rowIndex == fromRow) {
cell.getCTTc().addNewTcPr().addNewVMerge().setVal(org.apache.poi.xwpf.usermodel.STMerge.CONTINUE);
} else {
row.getCell(colIndex).getCTTc().addNewTcPr().addNewVMerge();
}
}
}
}
```
这个示例代码中,fillTableData 方法接收三个参数:文档模板路径、输出路径和需要填充的数据。在方法中,首先打开文档模板,然后获取需要填充数据的表格。接着,遍历数据,逐行填充表格。
在填充表格数据时,使用 getRowIndex 方法根据列名获取列索引,然后根据索引获取单元格,再填充单元格数据。如果单元格属于一个合并单元格,使用 getMergeRange 方法获取合并单元格的范围,并使用 mergeCellsVertically 方法将数据填充到合并单元格中。
最后,将修改后的文档保存到磁盘中。注意,在保存文档之前,需要先关闭文档对象。
相关推荐
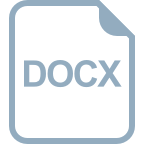














