C++省赛石头剪刀布
时间: 2025-01-01 15:30:42 浏览: 7
### C++ 实现石头剪刀布比赛代码
为了实现一个简单的石头剪刀布游戏,在C++中可以利用标准库中的随机数生成功能来模拟计算机的选择,并通过比较玩家和计算机的手势来决定胜负。
#### 头文件引入
首先,需要导入必要的头文件:
```cpp
#include <iostream>
#include <cstdlib> // srand, rand 函数
#include <ctime> // time(NULL) 函数
```
这些头文件提供了生成随机数以及获取当前时间的功能,用于初始化随机种子并让程序每次运行时都能有不同的结果[^1]。
#### 主要逻辑结构
定义手势对应的枚举类型以便于管理和判断输赢关系。接着编写函数处理用户的输入验证、电脑的选择过程及最终的结果判定。
```cpp
enum Gesture {
Rock,
Paper,
Scissors
};
// 将整数值转换成对应的手势名称字符串表示形式
std::string gestureToString(Gesture g) {
switch (g) {
case Rock: return "Rock";
case Paper: return "Paper";
case Scissors: return "Scissors";
default: return "";
}
}
int main() {
std::cout << "Let's play Rock-Paper-Scissors!" << std::endl;
// 初始化随机数发生器
srand(time(0));
while(true){
int userChoice;
do{
std::cout << "\nEnter your choice:\n"
<< "1 - Rock\n2 - Paper\n3 - Scissors\nYour turn: ";
std::cin >> userChoice;
}while(userChoice<1 || userChoice>3);
Gesture playerGesture = static_cast<Gesture>(userChoice - 1);
// 让电脑做出选择
Gesture computerGesture = static_cast<Gesture>(rand()%3);
std::cout << "You chose " << gestureToString(playerGesture)
<< ", and the Computer chose "
<< gestureToString(computerGesture) << "." << std::endl;
// 判断谁获胜
if ((playerGesture == Rock && computerGesture == Scissors)||
(playerGesture == Paper && computerGesture == Rock)||
(playerGesture == Scissors && computerGesture == Paper)){
std::cout << "Congratulations! You win this round." << std::endl;
}else if((computerGesture == Rock && playerGesture == Scissors)||
(computerGesture == Paper && playerGesture == Rock)||
(computerGesture == Scissors && playerGesture == Paper)){
std::cout << "Sorry, you lost this round." << std::endl;
} else {
std::cout << "It's a tie!" << std::endl;
}
char replay;
std::cout << "Do you want to play again? (y/n): ";
std::cin >> replay;
if(replay != 'y' && replay != 'Y') break;
}
return 0;
}
```
这段代码实现了基本的石头剪刀布游戏循环,允许用户多次挑战直到不想继续为止。它会提示用户输入选项,并显示双方的选择与胜利者的信息[^4]。
阅读全文
相关推荐








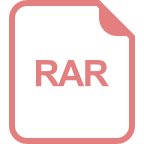







