pyqt excel插入图片时设置图片大小
时间: 2023-09-04 22:10:50 浏览: 122
可以使用QPixmap和QLabel等组件来实现在PyQt中插入图片并设置图片大小。以下是一个示例代码片段:
```python
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
# 创建QApplication
app = QApplication([])
# 创建主窗口
window = QMainWindow()
# 创建标签和图片
label = QLabel(window)
pixmap = QPixmap('image.png')
# 设置图片大小
pixmap = pixmap.scaled(100, 100) # 宽为100,高为100
# 在标签中设置图片
label.setPixmap(pixmap)
# 显示窗口
window.show()
# 运行程序
app.exec_()
```
在代码中,我们首先导入了PyQt5中的QPixmap和QLabel等组件。接下来,我们创建了一个QApplication和QMainWindow的实例来显示窗口。然后,我们创建了一个标签和一个QPixmap实例来加载要插入的图像。最后,我们使用scaled方法来调整图片的大小,并将其设置为标签的背景。
相关问题
pyqt excel插入图片insert_image设置大小
PyQt中使用QAxWidget组件可以实现在Excel中插入图片并设置图片大小的功能。具体的代码示例如下:
```
from PyQt5 import QtWidgets
from PyQt5.QtCore import QUrl
from PyQt5.QtWidgets import QApplication
from PyQt5.QAxContainer import QAxWidget
app = QApplication([])
myexcel = QAxWidget("Excel.Application")
myexcel.setProperty("Visible",True)
workbook = myexcel.querySubObject("Workbooks")
workbook_1 = workbook.Open("path_to_your.xlsx")
worksheet = workbook_1.Worksheets("Sheet1")
# 设置图片的路径
image_path = "path_to_your_image.jpg"
# 在单元格B2处插入图片
target_cell = worksheet.Range(worksheet.Cells(2,2), worksheet.Cells(2,2))
# 插入图片并设置大小
picture = worksheet.Shapes.AddPicture(image_path, False, True,
target_cell.Left, target_cell.Top, target_cell.Width, target_cell.Height)
myexcel.setProperty("Visible",False)
```
在代码中,我们先创建了一个QAxWidget对象,然后将其“Visible”属性设置为True,这样就可以在程序中看到Excel软件。接着,我们打开要插入图片的Excel文件,并定位到要插入图片的工作表。这里假设我们要把图片插入到Sheet1中的B2单元格。
接下来,我们指定要插入的图片文件路径,并将图片插入到目标单元格中。注意,在这里我们使用了Shapes.AddPicture()方法来插入图片,它的第二个参数表明了是否链接图片,第三个参数则表示是否保存与文档一起的副本。最后,我们将Excel软件从可见转为不可见,以便更好地与用户进行交互。
通过上述代码,就可以在PyQt中实现在Excel中插入图片并设置大小的功能了。
pyqt设置excel插入图片的大小
为了设置Excel插入图片的大小,可以使用如下代码:
```python
from PyQt5.QtCore import Qt
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
from win32com.client import Dispatch
from PIL import Image
# 创建Excel
excel = Dispatch("Excel.Application")
workbook = excel.Workbooks.Add()
worksheet = workbook.Worksheets("Sheet1")
# 插入图片
img_path = "image.png"
img = Image.open(img_path)
img_size = img.size
top_left_cell = worksheet.Range("A1")
width = img_size[0] / top_left_cell.Width * 3
height = img_size[1] / top_left_cell.Height * 3
worksheet.Shapes.AddPicture(img_path,
LinkToFile=False,
SaveWithDocument=True,
Left=top_left_cell.Left,
Top=top_left_cell.Top,
Width=int(width),
Height=int(height))
# 显示Excel界面
excel.Visible = True
```
其中,`width` 和 `height` 可以根据插入图片的大小进行调整。另外,需要注意的是,需要安装 `win32com` 和 `Pillow` 库。
阅读全文
相关推荐
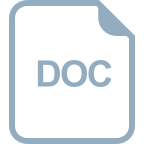
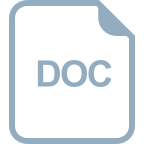
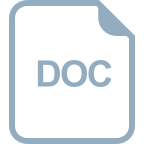










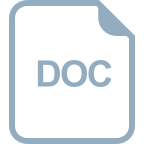
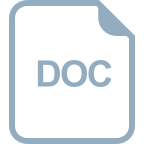