import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter; public class PrintWriterDemo { public static void main(String[] args) { try { File file = new File("output.txt"); PrintWriter pw = new PrintWriter(file); pw.println("Hello, PrintWriter!"); pw.println("This is a test."); pw.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } } }对以上JABA代码进行逐句解释
时间: 2024-02-14 10:10:58 浏览: 78
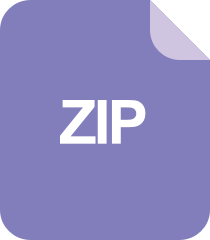
java源码:Java读写文本文件的示例代码.zip
好的,下面是对该Java代码逐句的解释:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class PrintWriterDemo {
public static void main(String[] args) {
try {
File file = new File("output.txt");
PrintWriter pw = new PrintWriter(file);
pw.println("Hello, PrintWriter!");
pw.println("This is a test.");
pw.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
- `import java.io.File;`:导入Java IO包中的File类,以便在程序中使用该类中的方法;
- `import java.io.FileNotFoundException;`:导入Java IO包中的FileNotFoundException异常类,以便在程序中处理文件未找到的异常;
- `import java.io.PrintWriter;`:导入Java IO包中的PrintWriter类,以便在程序中使用该类中的方法;
- `public class PrintWriterDemo {`:定义一个公共类PrintWriterDemo;
- `public static void main(String[] args) {`:定义main方法作为程序的入口;
- `try {`:开始try块;
- `File file = new File("output.txt");`:创建一个File对象file,该对象代表了名为output.txt的文件;
- `PrintWriter pw = new PrintWriter(file);`:创建一个PrintWriter对象pw,该对象将向文件output.txt中写入数据;
- `pw.println("Hello, PrintWriter!");`:向文件中写入一行字符串"Hello, PrintWriter!",并自动换行;
- `pw.println("This is a test.");`:向文件中写入一行字符串"This is a test.",并自动换行;
- `pw.close();`:关闭PrintWriter对象pw;
- `} catch (FileNotFoundException e) {`:捕获FileNotFoundException异常,并进入catch块;
- `e.printStackTrace();`:打印异常信息;
- `} catch (Exception e) {`:捕获其他异常,并进入catch块;
- `e.printStackTrace();`:打印异常信息;
- `}`:结束try-catch块。
阅读全文
相关推荐
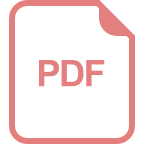
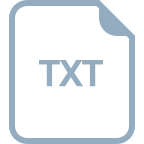















