解释这段代码package question7; import java.io.DataOutputStream; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.PrintWriter; import java.util.ArrayList; import java.util.HashSet; import java.util.Scanner; import java.util.Set; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.Random; public class Test { public static void main(String args[]){ Test test=new Test(); Scanner input=new Scanner(System.in); String s1=new String(); s1=input.nextLine(); String[] s2 = s1.split(" "); int[] l=new int[s2.length]; for(int i=0 ; i<s2.length;i++){ l[i]=Integer.parseInt(s2[i]); } test.write("test.txt", l); int[] readlist=test.read("test.txt",l); isPrime isprime=new isPrime(readlist); for(int i=1;i<=10;i++){ new Thread(isprime).start(); } try { Thread.sleep(1000); //1000 毫秒,也就是1秒. } catch(InterruptedException ex) { Thread.currentThread().interrupt(); } Set<Integer> set=new HashSet(); set=isprime.getSet(); System.out.println("素数set"+set); System.out.println("输入查询数字"); int num=input.nextInt(); if(set.contains(num)){ System.out.println(num+"是素数"); } else System.out.println(num+"不是是素数"); } public void write(String filename,int a[]){ File file = new File(filename); try { PrintWriter output=new PrintWriter(file); for(int i=0;i<a.length;i++){ output.print(a[i]+" "); } output.close(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public int[] read(String filename,int a[]){ File file=new File(filename); try { Scanner input=new Scanner(file); int i=0; while(input.hasNext()){ a[i]=input.nextInt(); i++; } } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } return a; } }
时间: 2023-06-02 20:08:12 浏览: 87
这段代码是一个 Java 程序,主要功能是读取用户输入的一串整数,将其写入文件 test.txt,然后又从这个文件中读取整数列表,并查找出其中的素数并存储在一个 HashSet 中,最后判断用户输入的数字是否为素数。具体实现包括定义了一个 isPrime 类用于判断一个数字是否为素数,使用了多线程来处理多个数字的判断,使用了 HashSet 来存储素数列表。
相关问题
請列出java.io內的函數
### 回答1:
java.io內的函數有:InputStream、OutputStream、Reader、Writer、File、FileDescriptor、Serializable、DataInput、DataOutput、Closeable、Flushable、FileFilter、FilenameFilter、FileInputStream、FileOutputStream、ObjectInputStream、ObjectOutputStream 以及 BufferedInputStream 等等。
### 回答2:
Java.io是Java编程语言中提供的类库之一,用于处理输入输出操作。在java.io包中,包含了许多类和函数。以下是一些常用的函数:
1. File类中的函数:
- createNewFile():创建一个新文件。
- delete():删除指定文件。
- exists():判断指定文件是否存在。
- getName():获取文件的名称。
- isDirectory():判断指定路径是否为目录。
- isFile():判断指定路径是否为文件。
- lastModified():获取文件最后修改的时间。
- length():获取文件的大小。
2. FileInputStream类中的函数:
- read():读取一个字节的数据。
- read(byte[]):将读取的数据存入指定的字节数组中。
- skip(long):跳过指定数量的字节。
- available():返回当前可读取的字节数。
- close():关闭输入流。
3. FileOutputStream类中的函数:
- write(int):写入一个字节的数据。
- write(byte[]):将指定字节数组中的数据写入文件。
- write(byte[], int, int):将指定字节数组的一部分写入文件。
- flush():刷新输出流缓冲区。
- close():关闭输出流。
4. BufferedReader类中的函数:
- readLine():读取一行文本。
- read():读取一个字符的数据。
- skip(long):跳过指定数量的字符。
- ready():检查是否可以从输入流中读取数据。
5. BufferedWriter类中的函数:
- write(int):写入一个字符的数据。
- write(String):将指定的字符串写入文件。
- newLine():写入一个行分隔符。
- flush():刷新输出流缓冲区。
- close():关闭输出流。
上述只是一些常用的java.io类中的函数,还有许多其他方法和功能,可以根据具体需求进行使用。
### 回答3:
Java.io是Java编程语言中用于处理输入和输出的标准包。这个包提供了一组用于读取和写入数据的类和接口。以下是java.io包中一些常用的函数:
1. File类:用于操作文件和目录。常用函数包括创建文件,删除文件,重命名文件,获取文件属性等。
2. FileInputStream和FileOutputStream类:用于读取和写入二进制数据。常用函数包括读取一个字节,读取多个字节,写入一个字节,写入多个字节等。
3. FileReader和FileWriter类:用于读取和写入文本数据。常用函数包括读取一个字符,读取多个字符,写入一个字符,写入多个字符等。
4. BufferedReader和BufferedWriter类:用于缓冲读取和写入数据。常用函数包括读取一行数据,写入一行数据等。
5. DataInputStream和DataOutputStream类:用于读取和写入基本数据类型。常用函数包括读取整数,读取浮点数,写入整数,写入浮点数等。
6. ObjectInputStream和ObjectOutputStream类:用于读取和写入对象。常用函数包括读取对象,写入对象等。
7. InputStreamReader和OutputStreamWriter类:用于将字节流转换成字符流。常用函数包括读取一个字符,写入一个字符等。
8. ZipInputStream和ZipOutputStream类:用于读取和写入ZIP格式的压缩文件。常用函数包括解压缩文件,压缩文件等。
以上仅仅是java.io包中的一部分函数,这些函数的功能和用法非常丰富,可以根据具体的需求选择适合的函数来进行输入和输出操作。
package socket; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import java.io.OutputStreamWriter; import java.net.ServerSocket; import java.net.Socket; public class SimpleTCPServer { public static void main(String[] args) throws IOException { ServerSocket server = new ServerSocket(8885); // 1、创建服务器,指定端口 while(true) { Socket socket = server.accept();// 2、接收客户端连接 阻塞式 Handler handler = new Handler(socket); handler.start(); } } } class Handler extends Thread{ private Socket socket; public Handler(Socket socket) { this.socket = socket; } public void run() { InputStream dis = null; OutputStream dos = null; System.out.println("线程名称:" + Thread.currentThread().getName()); try { dis = socket.getInputStream(); dos = socket.getOutputStream(); byte[] buf = new byte[1024]; int len = dis.read(buf); String msg = new String(buf,0,len); System.out.println("服务端从客户端接收到: " + msg); String echo = "欢迎"; dos.write(echo.getBytes()); // 3、发送数据 dos.flush(); } catch (IOException e) { e.printStackTrace(); } finally { try { dos.close(); dis.close(); socket.close(); } catch (IOException e) { e.printStackTrace(); } } } }
这是一个简单的TCP服务器端的Java代码,可以监听客户端连接并接收消息,然后发送欢迎消息。代码流程如下:
1. 创建ServerSocket对象,并指定端口号为8885;
2. 通过ServerSocket对象的accept()方法阻塞式地等待客户端连接,一旦有客户端连接则创建一个Handler对象处理该连接;
3. Handler类继承了Thread类,重写了run()方法,其中获取到了与客户端通信的InputStream和OutputStream对象,通过read()方法读取客户端发送来的数据,并将读取到的字节数据转换为字符串类型后输出到控制台;
4. 将欢迎消息转换为字节数组后通过write()方法发送给客户端,并调用flush()方法刷新输出流;
5. 在finally块中关闭数据输入流、数据输出流和Socket对象。
需要注意的是,这是一个单线程的服务器端程序,每次只能处理一个客户端连接,如果需要同时处理多个客户端连接,则需要使用多线程或NIO技术。
相关推荐
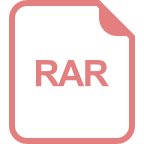
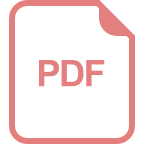
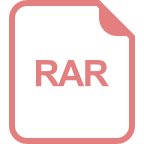












