使用c语言实现图的邻接表存储(无向图)
时间: 2023-11-05 07:15:39 浏览: 98
以下是使用 C 语言实现无向图邻接表存储的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int dest;
struct Node* next;
};
struct Graph {
int V;
struct Node** adjList;
};
struct Node* createNode(int dest) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
struct Graph* createGraph(int V) {
struct Graph* graph = (struct Graph*) malloc(sizeof(struct Graph));
graph->V = V;
graph->adjList = (struct Node**) malloc(V * sizeof(struct Node*));
for (int i = 0; i < V; i++) {
graph->adjList[i] = NULL;
}
return graph;
}
void addEdge(struct Graph* graph, int src, int dest) {
struct Node* newNode = createNode(dest);
newNode->next = graph->adjList[src];
graph->adjList[src] = newNode;
newNode = createNode(src);
newNode->next = graph->adjList[dest];
graph->adjList[dest] = newNode;
}
void printGraph(struct Graph* graph) {
for (int i = 0; i < graph->V; i++) {
struct Node* temp = graph->adjList[i];
printf("Adjacency list of vertex %d\nhead", i);
while (temp) {
printf(" -> %d", temp->dest);
temp = temp->next;
}
printf("\n");
}
}
int main() {
int V = 5;
struct Graph* graph = createGraph(V);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printGraph(graph);
return 0;
}
```
在上面的代码中,我们使用了两个结构体,一个是 `Node` 结构体表示邻接链表中的一个节点,另一个是 `Graph` 结构体表示整个图。
在 `createNode` 函数中,我们创建了一个新的邻接链表节点,并将其初始化为一个空节点。
在 `createGraph` 函数中,我们创建了一个新的图,并将其初始化为一个空图。
在 `addEdge` 函数中,我们将两个节点连接起来,因为这是一个无向图,所以我们需要在两个节点之间建立双向边。
在 `printGraph` 函数中,我们遍历整个图,并打印出每个节点的邻接链表。
在 `main` 函数中,我们首先创建了一个具有 5 个顶点的图,并添加了一些边。最后,我们打印图的邻接链表。
这就是使用 C 语言实现无向图邻接表存储的示例代码。
阅读全文
相关推荐






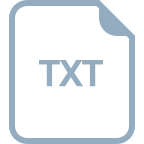
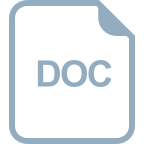







