用c语言实现邻接表存储
时间: 2023-10-21 15:07:12 浏览: 119
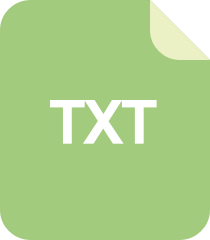
图的邻接表存储C语言实现
邻接表是一种图的存储结构,可以用来表示稀疏图。下面是一个用C语言实现邻接表存储的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct AdjListNode {
int dest;
struct AdjListNode* next;
};
struct AdjList {
struct AdjListNode* head;
};
struct Graph {
int V;
struct AdjList* array;
};
struct AdjListNode* newAdjListNode(int dest) {
struct AdjListNode* newNode = (struct AdjListNode*)malloc(sizeof(struct AdjListNode));
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
struct Graph* createGraph(int V) {
struct Graph* graph = (struct Graph*)malloc(sizeof(struct Graph));
graph->V = V;
graph->array = (struct AdjList*)malloc(V * sizeof(struct AdjList));
int i;
for (i = 0; i < V; ++i) {
graph->array[i].head = NULL;
}
return graph;
}
void addEdge(struct Graph* graph, int src, int dest) {
struct AdjListNode* newNode = newAdjListNode(dest);
newNode->next = graph->array[src].head;
graph->array[src].head = newNode;
newNode = newAdjListNode(src);
newNode->next = graph->array[dest].head;
graph->array[dest].head = newNode;
}
void printGraph(struct Graph* graph) {
int v;
for (v = 0; v < graph->V; ++v) {
struct AdjListNode* pCrawl = graph->array[v].head;
printf("\n Adjacency list of vertex %d\n head ", v);
while (pCrawl) {
printf("-> %d", pCrawl->dest);
pCrawl = pCrawl->next;
}
printf("\n");
}
}
int main() {
int V = 5;
struct Graph* graph = createGraph(V);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printGraph(graph);
return 0;
}
```
这个示例代码创建了一个包含5个顶点的无向图,然后使用邻接表存储这个图,并最终输出它的邻接表。在这个示例代码中,我们首先定义了三种结构体:`AdjListNode`、`AdjList`和`Graph`。其中,`AdjListNode`表示邻接表中的一个节点,包括目标节点的编号和指向下一个节点的指针;`AdjList`表示一个节点的邻接表,包括指向第一个节点的指针;`Graph`表示整个图,包括顶点数和一个指向邻接表数组的指针。
接下来,我们定义了一些函数来操作这些结构体,包括`newAdjListNode`用于创建一个新的邻接表节点,`createGraph`用于创建一个新的图,`addEdge`用于向图中添加一条边,`printGraph`用于输出图的邻接表。
在`main`函数中,我们首先创建了一个包含5个顶点的图,然后添加了7条边,最后输出了这个图的邻接表。
以上就是一个简单的使用C语言实现邻接表存储的示例代码。
阅读全文
相关推荐















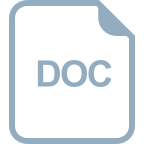