String - count the frequency of characters in a string Given a string S, count the frequency of characters in the string S (the occurrences of a character present in the string) Input: hello world where: First-line represents the input string Output: d 1 e 1 h 1 l 3 o 2 r 1 w 1 Explanation: Print the count of each distinct letter present in string S. Assumptions: String contains only lower case letters Length of string S can be 0 to 10000
时间: 2023-11-27 09:51:37 浏览: 34
Here's a possible solution in Java:
```java
import java.util.HashMap;
import java.util.Map;
public class CharacterFrequency {
public static void main(String[] args) {
String s = "hello world";
Map<Character, Integer> frequency = new HashMap<>();
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
frequency.put(c, frequency.getOrDefault(c, 0) + 1);
}
for (Map.Entry<Character, Integer> entry : frequency.entrySet()) {
System.out.println(entry.getKey() + " " + entry.getValue());
}
}
}
```
The `frequency` map stores the count of each distinct character in the string. We loop through the string `s`, and for each character `c`, we update its count in the map using `frequency.put(c, frequency.getOrDefault(c, 0) + 1)`. The `getOrDefault` method returns the current count of `c` in the map, or 0 if it's not present yet, and we add 1 to it. Finally, we loop through the entries of the `frequency` map and print each character and its count.
Output:
```
d 1
e 1
h 1
l 3
o 2
r 1
w 1
```
相关推荐
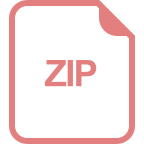
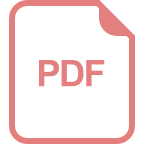















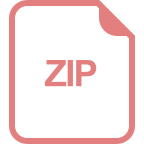