c语言 结构体指针传值
时间: 2023-09-24 11:13:05 浏览: 157
在C语言中,可以通过结构体指针来传递结构体的值。结构体指针允许我们传递结构体的引用,而不是实际的结构体对象。
下面是一个示例,演示了如何使用结构体指针传递值:
```c
#include <stdio.h>
typedef struct {
int id;
char name[20];
} Student;
void printStudent(Student *ptr) {
printf("ID: %d\n", ptr->id);
printf("Name: %s\n", ptr->name);
}
int main() {
Student s;
s.id = 1;
strcpy(s.name, "Alice");
printStudent(&s);
return 0;
}
```
在上面的示例中,定义了一个名为`Student`的结构体类型。然后,在`printStudent`函数中,接受一个指向`Student`结构体的指针作为参数。在`main`函数中,创建一个`Student`类型的变量`s`,并将其地址传递给`printStudent`函数。
在`printStudent`函数中,通过指针访问结构体的成员,使用箭头操作符`->`来访问结构体成员。
通过结构体指针传递值可以避免复制大型结构体的开销,并且可以在函数内部修改结构体的值。
相关问题
C语言怎么用结构体传值给别的函数
在C语言中,由于传递结构体是按值传递(value copy),即函数接收到的是结构体的一个副本而不是原结构体本身,所以直接将结构体作为参数传入函数时,函数内的修改不会影响到原始的结构体。如果你想让函数改变结构体的内容,你可以这样做:
1. **通过指针传递**:将结构体的地址(也就是指针)作为函数参数,这样函数就可以操作这个指向实际结构体的内存了。
```c
void modify(Student *s) {
s->age = 20; // 修改结构体s的age字段
}
int main() {
Student stu = {18, "Alice", 95};
modify(&stu); // 将stu的地址传给modify函数
printf("stu.age = %d\n", stu.age); // 输出:stu.age = 20
}
```
2. **使用引用传递(仅C++支持)**:在C++中,可以使用`&`运算符获取结构体引用,类似于指针,但是提供更强的类型安全性和更简洁的语法。
```cpp
// 在C++中
void modify(Student &s) {
s.age = 20; // 直接修改引用所指向的结构体内容
}
// 主函数同上
```
C语言实参形参传值传地址的区别,多举几个例子
在C语言中,实参(实际参数)和形参(形式参数)之间的传递方式主要有两种:传值和传地址。这两种方式有显著的区别。
### 1. 传值(Pass by Value)
传值是指将实参的值复制一份传递给形参。形参在函数内部的修改不会影响到实参。
#### 示例:
```c
#include <stdio.h>
void modifyValue(int x) {
x = 10;
}
int main() {
int a = 5;
modifyValue(a);
printf("a = %d\n", a); // 输出: a = 5
return 0;
}
```
在这个例子中,`modifyValue`函数接收一个整型参数`x`,并将其值修改为10。然而,由于是传值调用,函数内部的修改不会影响到`main`函数中的变量`a`。
### 2. 传地址(Pass by Reference)
传地址是指将实参的地址传递给形参。通过地址,形参可以访问并修改实参的值。
#### 示例:
```c
#include <stdio.h>
void modifyValue(int *x) {
*x = 10;
}
int main() {
int a = 5;
modifyValue(&a);
printf("a = %d\n", a); // 输出: a = 10
return 0;
}
```
在这个例子中,`modifyValue`函数接收一个整型指针参数`x`,并通过解引用操作符`*`修改`x`指向的值为10。由于是传地址调用,函数内部的修改会直接影响到`main`函数中的变量`a`。
### 3. 传结构体(Passing Structs)
传结构体时,既可以传值也可以传地址。
#### 传值示例:
```c
#include <stdio.h>
struct Point {
int x;
int y;
};
void modifyPoint(struct Point p) {
p.x = 10;
p.y = 20;
}
int main() {
struct Point p1 = {1, 2};
modifyPoint(p1);
printf("p1.x = %d, p1.y = %d\n", p1.x, p1.y); // 输出: p1.x = 1, p1.y = 2
return 0;
}
```
#### 传地址示例:
```c
#include <stdio.h>
struct Point {
int x;
int y;
};
void modifyPoint(struct Point *p) {
p->x = 10;
p->y = 20;
}
int main() {
struct Point p1 = {1, 2};
modifyPoint(&p1);
printf("p1.x = %d, p1.y = %d\n", p1.x, p1.y); // 输出: p1.x = 10, p1.y = 20
return 0;
}
```
在传结构体时,传地址可以避免复制整个结构体,提高效率,并且可以修改结构体的内容。
### 总结
- **传值**:复制实参的值,形参的修改不会影响实参。
- **传地址**:传递实参的地址,形参可以通过地址访问并修改实参的值。
阅读全文
相关推荐









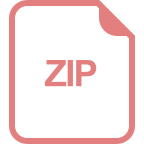




