用javaSSM框架编程题:现有学生类和班级类 学生:具有学号、姓名、年龄和班级属性,以及一个 sayHello() 方法 班级:班级号 班级名称 请通过Spring依赖注入的方式,调用学生的sayHello()方法在控制台输出学生的全部类里面的信息。
时间: 2024-09-13 09:06:43 浏览: 43
在Java的SSM(Spring + Spring MVC + MyBatis)框架中,要实现学生类(Student)和班级类(Class)的依赖注入,并调用学生的sayHello()方法输出信息,需要经过以下步骤:
1. 定义Student类和Class类。Student类包含学号、姓名、年龄和Class属性,以及sayHello()方法。Class类包含班级号和班级名称。
```java
public class Student {
private String studentId;
private String name;
private int age;
private Class clazz;
// 构造方法、getter和setter省略
public void sayHello() {
System.out.println("学号:" + studentId + ", 姓名:" + name + ", 年龄:" + age + ", 班级:" + clazz.getClassName());
}
}
public class Class {
private String classId;
private String className;
// 构造方法、getter和setter省略
}
```
2. 创建Spring的配置文件(applicationContext.xml),配置Student和Class的bean,并通过setter注入的方式注入Class对象到Student对象。
```xml
<beans ...>
<!-- 配置Class bean -->
<bean id="classBean" class="com.example.Class">
<property name="classId" value="101"/>
<property name="className" value="计算机科学与技术"/>
</bean>
<!-- 配置Student bean -->
<bean id="studentBean" class="com.example.Student">
<property name="studentId" value="S001"/>
<property name="name" value="张三"/>
<property name="age" value="20"/>
<!-- 依赖注入Class对象 -->
<property name="clazz" ref="classBean"/>
</bean>
</beans>
```
3. 创建Spring的测试类,获取Student的bean并调用sayHello()方法。
```java
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestSSM {
public static void main(String[] args) {
// 加载Spring配置文件
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
// 通过Spring容器获取Student的bean
Student student = (Student) context.getBean("studentBean");
// 调用sayHello()方法输出学生信息
student.sayHello();
}
}
```
运行TestSSM类的main方法,控制台将输出学生的所有信息。
相关推荐
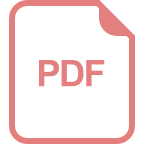
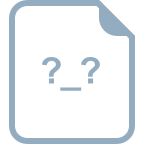
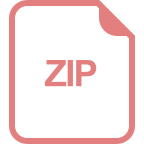














