设计一个学生结构体,包括学号、3门课成绩及总成绩。输入n个学生的学号及3门课的成绩,计算每个学生的总成绩,并输出。 输入格式: 先输入n,再输入3门课成绩(每门成绩有1位小数),每行1位学生。 输出格式: 输出学生的学号及总成绩,按照总成绩从高到底排序,每行1位学生。
时间: 2024-03-23 16:42:44 浏览: 61
好的,这是一个结构体和排序的问题。以下是示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_N 50
#define MAX_ID_LEN 10
struct Student {
char id[MAX_ID_LEN];
float score[3];
float total_score;
};
int cmp(const void *a, const void *b) {
struct Student *s1 = (struct Student *)a;
struct Student *s2 = (struct Student *)b;
return s2->total_score - s1->total_score;
}
int main() {
int n;
struct Student students[MAX_N];
scanf("%d", &n);
for (int i = 0; i < n; i++) {
scanf("%s %f %f %f", students[i].id, &students[i].score[0], &students[i].score[1], &students[i].score[2]);
students[i].total_score = students[i].score[0] + students[i].score[1] + students[i].score[2];
}
// 使用快速排序按总成绩从高到低排序
qsort(students, n, sizeof(struct Student), cmp);
// 输出结果
for (int i = 0; i < n; i++) {
printf("%s %.1f\n", students[i].id, students[i].total_score);
}
return 0;
}
```
这段代码定义了一个结构体 `Student`,包括学号、3门课成绩及总成绩。然后读入每个学生的信息,计算总成绩,并使用 `qsort` 函数按总成绩从高到低排序。最后输出排序后的结果。注意,在 `qsort` 函数中定义了一个比较函数 `cmp`,用于比较两个学生的总成绩大小。
阅读全文
相关推荐
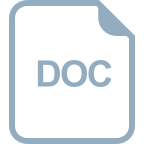















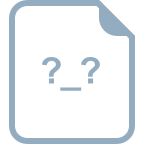