C语言编写函数,显示字母c前后的n个字符,中间用一个空格隔开,大小写不变。在主函数中输入字符c和正整数n。例如: 输入: D 2 显示:BC EF 输入:d 2 显示:bc ef 字母表看作首尾相接的。n可能会大于26。
时间: 2024-05-08 21:16:55 浏览: 40
```c
#include <stdio.h>
void showChar(char c, int n) {
// 将字母转换为大写,方便后续处理
if (c >= 'a' && c <= 'z') {
c -= 32;
}
// 计算前面 n 个字符
for (int i = 1; i <= n; i++) {
char ch = c - i;
if (ch < 'A') {
ch += 26;
}
printf("%c ", ch);
}
// 输出 c
printf("%c ", c);
// 计算后面 n 个字符
for (int i = 1; i <= n; i++) {
char ch = c + i;
if (ch > 'Z') {
ch -= 26;
}
printf("%c ", ch);
}
}
int main() {
char c;
int n;
scanf("%c %d", &c, &n);
showChar(c, n);
return 0;
}
```
相关问题
编写函数,显示字母c前后的n个字符,中间用一个空格隔开,大小写不变。在主函数中输入字符c和正整数n。用c语言
实现。
```c
#include <stdio.h>
void showChars(char c, int n) {
int i;
for (i = -n; i <= n; i++) {
if (i == 0) {
printf(" ");
} else {
printf("%c", c + i);
}
}
}
int main() {
char c;
int n;
printf("Enter a character and a positive integer: ");
scanf("%c%d", &c, &n);
printf("The characters are: ");
showChars(c, n);
printf("\n");
return 0;
}
```
2.写函数,将一个字符串中的元音字母复制到另一个字符串,然后输出。ψ 3.编写函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其它字符的个数,在主函数中输入字符串以及输出上述结果。4 4.写函数用起泡法对输入的10个字符串按由小到大的顺序排列。用c语言编写
2. 下面是一个 C 语言的实现,将字符串中的元音字母复制到另一个字符串并输出:
```c
#include <stdio.h>
#include <string.h>
void copy_vowels(char* input_string) {
char vowels[] = "aeiouAEIOU";
char result[100];
int len = strlen(input_string);
int j = 0;
for (int i = 0; i < len; i++) {
if (strchr(vowels, input_string[i])) {
result[j] = input_string[i];
j++;
}
}
result[j] = '\0';
printf("%s\n", result);
}
```
你可以调用这个函数,并将需要处理的字符串作为参数传入,例如:
```c
char input_string[] = "Hello World!";
copy_vowels(input_string);
```
这将输出:
```
eoO
```
3. 下面是一个 C 语言的实现,统计字符串中字母、数字、空格和其他字符的个数:
```c
#include <stdio.h>
#include <ctype.h>
void count_chars(char* input_string) {
int letters = 0;
int digits = 0;
int spaces = 0;
int others = 0;
int len = strlen(input_string);
for (int i = 0; i < len; i++) {
if (isalpha(input_string[i])) {
letters++;
} else if (isdigit(input_string[i])) {
digits++;
} else if (isspace(input_string[i])) {
spaces++;
} else {
others++;
}
}
printf("Letters: %d\n", letters);
printf("Digits: %d\n", digits);
printf("Spaces: %d\n", spaces);
printf("Others: %d\n", others);
}
```
你可以调用这个函数,并将需要处理的字符串作为参数传入,例如:
```c
char input_string[] = "Hello World! 123";
count_chars(input_string);
```
这将输出:
```
Letters: 10
Digits: 3
Spaces: 2
Others: 1
```
4. 下面是一个 C 语言的实现,使用冒泡排序对 10 个字符串按从小到大的顺序进行排列:
```c
#include <stdio.h>
#include <string.h>
void bubble_sort(char strings[][100], int n) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (strcmp(strings[j], strings[j+1]) > 0) {
char temp[100];
strcpy(temp, strings[j]);
strcpy(strings[j], strings[j+1]);
strcpy(strings[j+1], temp);
}
}
}
}
int main() {
char strings[10][100] = {
"banana",
"apple",
"pear",
"orange",
"watermelon",
"grape",
"lemon",
"peach",
"kiwi",
"mango"
};
bubble_sort(strings, 10);
for (int i = 0; i < 10; i++) {
printf("%s ", strings[i]);
}
printf("\n");
return 0;
}
```
这将输出:
```
apple banana grape kiwi lemon mango orange peach pear watermelon
```
阅读全文
相关推荐
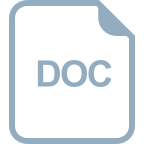
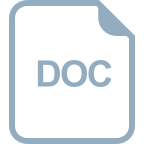
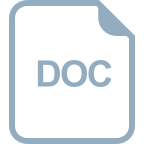














