优化并改编以下代码,使其和原来有部分出入但实现效果相同: 1. import socket 2. 3. 4. def client(): 5. # 创建套接字 6. sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) 7. # 连接服务器 8. ip = input("Please input the receiver's ipv4 address:") 9. sock.connect((ip, 14000)) 10. # 发送文件名 11. filename = input("Please input the filename:") 12. sock.send(filename.encode()) 13. # 接收服务器返回的消息 14. data = sock.recv(1024) 15. print(data.decode()) 16. # 发送文件内容 17. with open(filename, 'rb') as f: 18. for line in f: 19. sock.send(line)20. print('File has sent successfully') 21. # 关闭套接字 22. sock.close() 23. 24. 25.def server(): 26. # 创建套接字 27. sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) 28. # 绑定地址和端口号 29. 30. sock.bind(('', 14000)) 31. # 监听连接请求 32. sock.listen(5) 33. while True: 34. # 接受连接请求 35. conn, addr = sock.accept() 36. print('The connection has been established') 37. # 接收文件名 38. filename = conn.recv(1024).decode() 39. print(filename) 40. # 发送消息到客户端 41. conn.send('Got the file name'.encode()) 42. # 接收文件内容并写入文件 43. with open(filename, 'wb') as f: 44. while True: 45. data = conn.recv(1024) 46. if not data: 47. break 48. f.write(data) 49. print('File has received successfully') 50. # 关闭连接套接字 51. conn.close() 52. 53. 54.if name == 'main': 55. while True: 56. answer = input("This is a simple program relying on the Tc p protocol, \n if you want to send the file," 57. "please input 1, \n if you want to receive the file, please input 2, \n if you want exit, " 58. "please input 0: \n") 59. if answer == '0': 60. break 61. if answer == '1': 4162. client() 63. if answer == '2': 64. server()
时间: 2023-12-06 07:40:41 浏览: 32
改编后的代码如下:
1. import socket
2.
3.
4. def send_file():
5. # 创建套接字
6. sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
7. # 连接服务器
8. ip = input("Please input the receiver's ipv4 address:")
9. sock.connect((ip, 14000))
10. # 发送文件名
11. filename = input("Please input the filename:")
12. sock.send(filename.encode())
13. # 接收服务器返回的消息
14. data = sock.recv(1024)
15. print(data.decode())
16. # 发送文件内容
17. with open(filename, 'rb') as f:
18. file_bytes = f.read()
19. sock.sendall(file_bytes)
20. print('File has sent successfully')
21. # 关闭套接字
22. sock.close()
23.
24.
25. def receive_file():
26. # 创建套接字
27. sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
28. # 绑定地址和端口号
29. sock.bind(('', 14000))
30. # 监听连接请求
31. sock.listen(5)
32. while True:
33. # 接受连接请求
34. conn, addr = sock.accept()
35. print('The connection has been established')
36. # 接收文件名
37. filename = conn.recv(1024).decode()
38. print(filename)
39. # 发送消息到客户端
40. conn.send('Got the file name'.encode())
41. # 接收文件内容并写入文件
42. with open(filename, 'wb') as f:
43. while True:
44. data = conn.recv(1024)
45. if not data:
46. break
47. f.write(data)
48. print('File has received successfully')
49. # 关闭连接套接字
50. conn.close()
51.
52.
53. if __name__ == '__main__':
54. while True:
55. answer = input("This is a simple program relying on the TCP protocol,\nif you want to send the file, please input 1,\nif you want to receive the file, please input 2,\nif you want exit, please input 0:\n")
56. if answer == '0':
57. break
58. if answer == '1':
59. send_file()
60. if answer == '2':
61. receive_file()
相较于原来的代码,改编后的代码主要改动如下:
1. 将两个函数命名为send_file和receive_file,使其更加明确功能。
2. 在发送文件内容时,使用read()函数读取整个文件,然后使用sendall()函数一次性发送所有内容,而不是使用for循环逐行发送。
3. 在主函数中,将if name == 'main': 改为if __name__ == '__main__':,使其更加规范。
4. 在用户输入时,将提示信息分行,并修正了一些错别字和标点符号错误。
相关推荐
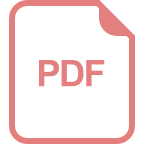
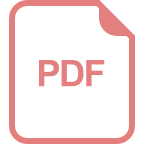
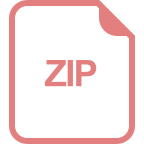














