从屏幕输入一段英文文字,统计其中出现的英文单词及其出现次数。(字典)要求程序可以过滤掉常见的标点符号,并按下面要求输出: (1)将出现次数大于2的单词按字典序输出并输出其出现次数。 (2)将出现次数大于2的单词按单词出现次数从大到小的排序输出,并输出其出现次数。
时间: 2024-05-12 19:19:58 浏览: 68
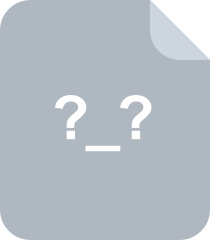
统计文章单词数并按字典序输出

由于本题需要使用字典来统计单词出现次数,因此我们可以使用 Python 中的 defaultdict 类来实现。具体步骤如下:
1. 从屏幕输入一段英文文字,并将其中的标点符号过滤掉。这可以通过使用 string 模块中的 punctuation 常量来实现。
2. 将过滤后的文本按空格分割成单词列表。
3. 遍历单词列表,对每个单词进行统计。统计时需要注意将单词转换为小写,以避免大小写造成的重复计数。
4. 将统计结果按字典序和出现次数排序,并输出。
下面是代码实现:
```python
import string
from collections import defaultdict
text = input('请输入一段英文文字:')
# 过滤标点符号
for punctuation in string.punctuation:
text = text.replace(punctuation, ' ')
# 分割单词
words = text.split()
# 统计单词出现次数
word_count = defaultdict(int)
for word in words:
word_count[word.lower()] += 1
# 按字典序排序并输出出现次数大于2的单词
for word in sorted(filter(lambda x: word_count[x] > 2, word_count)):
print(word, word_count[word])
# 按出现次数排序并输出出现次数大于2的单词
for word in sorted(word_count, key=lambda x: (-word_count[x], x)):
if word_count[word] > 2:
print(word, word_count[word])
```
这里使用了 lambda 表达式来过滤出现次数大于2的单词,并对出现次数进行降序排序。由于 Python 中的排序默认是升序,因此可以通过对出现次数取负来实现降序排序。
阅读全文
相关推荐
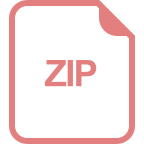
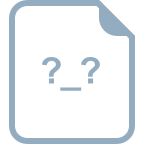





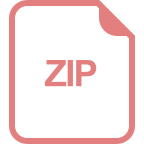
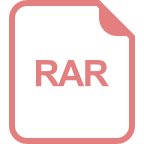
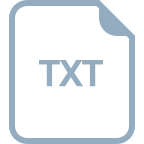
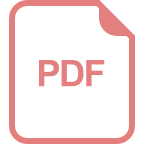
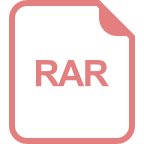
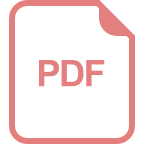
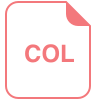
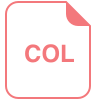
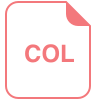
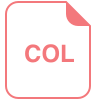
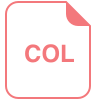