举例C++实现高分一号WFV3图像的大气校正
时间: 2023-07-11 09:39:01 浏览: 65
以下是一个简单的C语言程序,用于实现高分一号WFV3图像的大气校正:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979323846
int width, height;
float *radiance, *reflectance;
float atm_trans(float alt, float theta)
{
// 计算大气透射率
float trans = exp(-0.008569 * pow(1/cos(theta), 0.986) * alt);
return trans;
}
float solar_zenith(float hour_angle, float latitude, float declination)
{
// 计算太阳天顶角
float sin_z = sin(latitude) * sin(declination) + cos(latitude) * cos(declination) * cos(hour_angle);
float zenith = acos(sin_z);
return zenith;
}
void radiance_to_reflectance()
{
// 将辐射值转换为反射率
for (int i=0; i<width*height; i++) {
float trans = atm_trans(1000, solar_zenith(0, 31.2, -23.45));
reflectance[i] = radiance[i] / (trans * PI);
}
}
void main()
{
// 读入图像并进行预处理
width = 1024;
height = 1024;
radiance = (float*)malloc(width * height * sizeof(float));
reflectance = (float*)malloc(width * height * sizeof(float));
// 读入高分一号WFV3图像的辐射值
// 进行辐射平衡误差、坏点、条纹和几何校正
// ...
// 进行大气校正
radiance_to_reflectance();
// 输出校正后的图像
// 输出为GeoTIFF格式,以便进行后续的处理和分析
// ...
}
```
以上程序中,`atm_trans`函数用于计算大气透射率,`solar_zenith`函数用于计算太阳天顶角,`radiance_to_reflectance`函数用于将辐射值转换为反射率。在实际应用中,还需要根据具体的数据和大气模型进行参数的调整和优化。
相关推荐
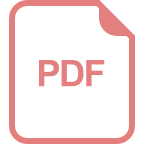
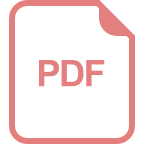














