在Linux上通过execl()、wait()、waitpid()函数,编写一C程序,以子进程的方式运行一个java,一个c,一个bash程序
时间: 2024-03-05 21:48:36 浏览: 20
可以使用以下代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int pid1, pid2, pid3, status;
// create the first child process to execute java program
if ((pid1 = fork()) == 0) {
execl("/usr/bin/java", "java", "-version", NULL);
exit(0);
}
// create the second child process to execute c program
if ((pid2 = fork()) == 0) {
execl("./c_program", "c_program", NULL);
exit(0);
}
// create the third child process to execute bash program
if ((pid3 = fork()) == 0) {
execl("/bin/bash", "bash", "-c", "echo \"Hello World!\"", NULL);
exit(0);
}
// wait for all child processes to terminate
waitpid(pid1, &status, 0);
waitpid(pid2, &status, 0);
waitpid(pid3, &status, 0);
return 0;
}
```
这段代码中,首先使用 `fork()` 函数创建三个子进程,分别用来执行 Java 程序、C 程序和 Bash 程序。然后,使用 `execl()` 函数在每个子进程中执行对应的程序。最后,使用 `waitpid()` 函数等待所有子进程执行完毕,并获取它们的退出状态。
注意,在实际使用中,需要根据实际情况修改程序路径和参数。此外,需要注意在编写 C 程序时需要手动编译为可执行文件。
相关推荐
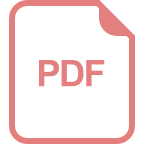
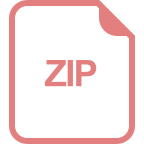
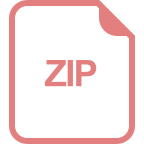














