对字符串和Long怎么判断非空
时间: 2023-05-31 18:06:28 浏览: 207
判断字符串非空可以使用以下方法:
1. 使用String类的isEmpty()方法来判断字符串是否为空,如果返回true,则表示字符串为空。
2. 使用String类的length()方法来获取字符串的长度,如果长度为0,则表示字符串为空。
3. 使用StringUtils类的isNotBlank()方法来判断字符串是否为空,如果返回true,则表示字符串非空。
判断Long非空可以使用以下方法:
1. 使用Long类的valueOf()方法将字符串转换成Long类型,如果字符串为空或无法转换成Long类型,则会抛出NumberFormatException异常。
2. 使用Long类的equals()方法来判断Long对象是否为null,如果为null,则表示Long对象为空。
3. 使用Java 8的Optional类来处理可能为空的Long对象,可以使用Optional.ofNullable()方法来创建Optional对象,然后使用isPresent()方法来判断是否为空。
相关问题
要求:从键盘输入一个小括号组成的字符串,判断该字符串中的括号是否匹配 解题思路: 1、定义一个字符数组kh[21],存储从键盘输入的括号字符串 提醒:字符串存储到字符数组中时,结束标记是‘\0’ 2、定义一个指针p,指向字符数组,当p指向的元素是‘(’,‘(’入栈;当p指向的元素是‘)’,‘(’出栈; 3、重复执行第2步,直到字符串遍历结束或者是栈变成空栈; 4、结果1:字符串遍历结束但是栈非空,说明‘(’多于‘)’; 5、结果2:字符串遍历没有结束但是栈为空,说明‘)’多于‘(’; 6、结果3:字符串遍历结束并且栈为空,说明括号是匹配的
以下是一个示例代码,实现了输入小括号组成的字符串并判断括号是否匹配:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义栈结构体
typedef struct {
char data[21]; // 存储括号的字符数组
int top; // 栈顶指针
} Stack;
// 初始化栈
void init(Stack *s) {
s->top = -1;
}
// 入栈
void push(Stack *s, char c) {
if (s->top == 20) {
printf("Stack is full!\n");
exit(1);
}
s->data[++(s->top)] = c;
}
// 出栈
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack is empty!\n");
exit(1);
}
return s->data[(s->top)--];
}
int main() {
char kh[21]; // 存储括号的字符数组
Stack s; // 定义栈
char *p = kh; // 指针p指向字符数组kh
int i = 0; // 计数器
printf("Please input the string of parentheses (no more than 20):\n");
scanf("%s", kh); // 输入括号字符串
init(&s); // 初始化栈
while (*p != '\0') { // 遍历字符数组
if (*p == '(') {
push(&s, *p); // 左括号入栈
} else if (*p == ')') {
if (s.top == -1) {
printf("Parentheses are not matched!\n");
exit(1);
} else {
pop(&s); // 右括号出栈
}
}
p++; // 指针p移动到下一个字符
i++; // 计数器加1
}
if (s.top == -1 && i <= 20) {
printf("Parentheses are matched!\n");
} else if (s.top != -1) {
printf("Parentheses are not matched!\n");
} else {
printf("Input string is too long!\n");
}
return 0;
}
```
在代码中,我们使用了一个栈结构体来存储左括号,当遍历到右括号时,如果栈非空,则出栈一个左括号,否则说明括号不匹配。最终,根据栈是否为空和输入字符串的长度来判断括号是否匹配。
给定一个整数Q(2<=Q<=10),一个非空字符串,以及另一个整数T(2<=T<=10), 编程要求过滤掉字符串中所有非Q进制数对应的字符组成一个新的字符串,该字符串无正负号,将该字符串表示的Q进制数转换为T进制数的字符串输出。 用C语言解答
在C语言中,你可以通过以下几个步骤来解决这个问题:
1. 定义函数`filterAndConvert`接收输入的三个参数:整数Q、非空字符串str和整数T。
```c
#include <stdio.h>
#include <string.h>
// 函数用于过滤并转换字符串
char* filterAndConvert(int Q, const char *str, int T) {
// 初始化变量
size_t str_len = strlen(str);
char filteredStr[str_len];
int qBaseIndex = 0;
for (size_t i = 0; i < str_len; ++i) {
if (isdigit(str[i]) && str[i] >= '0' && str[i] <= '9') { // 检查字符是否是非Q进制数字
filteredStr[qBaseIndex++] = str[i] - '0'; // 过滤并存储
}
}
filteredStr[qBaseIndex] = '\0'; // 添加字符串结束符
// 将过滤后的字符串转换为Q进制整数
long long int numInQ = strtoll(filteredStr, NULL, Q);
// 将Q进制整数转换为T进制字符串
char*tbaseResult = malloc(sizeof(char)*(T + 1));
sprintf(tbaseResult, "%lld", numInQ); // 使用sprintf而不是printf防止溢出
tbaseResult[strlen(tbaseResult)-1] = '\0'; // 去除末尾多余的'0'
return tbaseResult;
}
```
2. 主函数中获取用户输入并调用上述函数:
```c
int main() {
int Q, T;
scanf("%d %d", &Q, &T);
char str[100];
fgets(str, sizeof(str), stdin); // 注意fgets处理换行符
str[strcspn(str, "\n")] = '\0'; // 移除末尾的换行符
char* result = filterAndConvert(Q, str, T);
printf("转换后的T进制数为: %s\n", result);
free(result); // 释放内存
return 0;
}
阅读全文
相关推荐




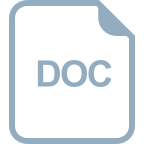












