rsa加密算法java实现
时间: 2023-07-26 16:12:23 浏览: 116
RSA加密算法的Java实现需要用到Java Cryptography Architecture (JCA)和Java Cryptography Extension (JCE)库。下面是一个简单的RSA加密算法Java实现的示例代码:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import javax.crypto.Cipher;
public class RSAEncryption {
private static final int KEY_SIZE = 2048;
public static void main(String[] args) throws Exception {
String plainText = "Hello, world!";
KeyPair keyPair = generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
byte[] encryptedBytes = encrypt(plainText, publicKey);
String encryptedText = new String(encryptedBytes);
System.out.println("Encrypted text: " + encryptedText);
byte[] decryptedBytes = decrypt(encryptedBytes, privateKey);
String decryptedText = new String(decryptedBytes);
System.out.println("Decrypted text: " + decryptedText);
}
private static KeyPair generateKeyPair() throws Exception {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(KEY_SIZE);
return keyPairGenerator.generateKeyPair();
}
private static byte[] encrypt(String plainText, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(plainText.getBytes());
}
private static byte[] decrypt(byte[] encryptedBytes, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(encryptedBytes);
}
}
```
在这个例子中,我们使用2048位的RSA密钥对生成器生成公钥和私钥。然后,我们使用公钥加密明文,使用私钥解密密文。注意,在实际应用中,我们应该将密文转换为Base64字符串以便传输。
阅读全文
相关推荐







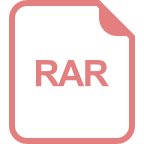




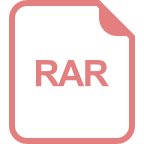
