jgit git.add
时间: 2023-10-13 17:23:14 浏览: 122
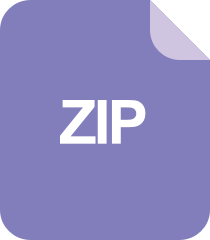
gradle-git-properties:用于`git.properties` 文件生成的 Gradle 插件

`jgit` 是一个用于 Java 编程语言的 Git 库。`git.add` 是 jgit 库中的一个方法,用于将文件或目录添加到 Git 版本控制中。
下面是一个使用 jgit 的示例代码,将文件添加到 Git 中:
```java
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
import java.io.File;
public class JGitAddExample {
public static void main(String[] args) {
try (Git git = Git.init().setDirectory(new File("/path/to/repo")).call()) {
git.add().addFilepattern("file.txt").call();
} catch (GitAPIException e) {
e.printStackTrace();
}
}
}
```
这段代码将 `file.txt` 文件添加到 `/path/to/repo` 目录中的 Git 版本控制中。
阅读全文
相关推荐
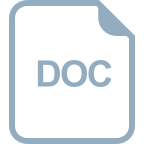
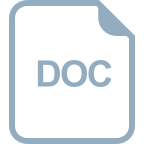
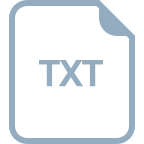
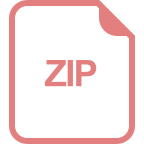
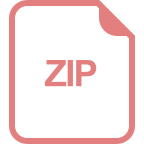
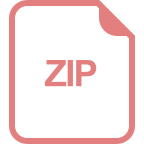
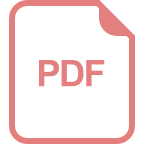
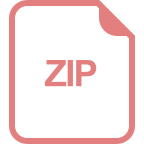
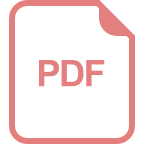
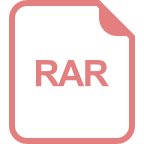
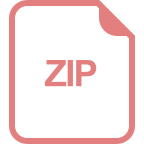
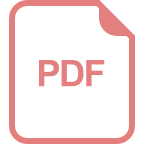
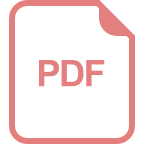




