RANSAC筛选和仿射变换实现图像间的坐标对齐c++
时间: 2024-02-25 07:55:01 浏览: 70
RANSAC(Random Sample Consensus)算法是一种常用的模型估计算法,可以用于筛选出在数据集中符合某个模型的数据点,并用这些数据点去估计模型参数。在图像处理中,RANSAC算法可以用于筛选出在两幅图像中匹配的特征点,并用这些特征点去估计图像之间的仿射变换矩阵,从而实现图像间的坐标对齐。
以下是实现图像间坐标对齐的C++代码:
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// 读入两张待对齐的图像
Mat image1 = imread("image1.jpg");
Mat image2 = imread("image2.jpg");
// 提取图像特征点
Ptr<FeatureDetector> detector = ORB::create();
vector<KeyPoint> keypoints1, keypoints2;
detector->detect(image1, keypoints1);
detector->detect(image2, keypoints2);
// 计算特征点的描述子
Ptr<DescriptorExtractor> extractor = ORB::create();
Mat descriptors1, descriptors2;
extractor->compute(image1, keypoints1, descriptors1);
extractor->compute(image2, keypoints2, descriptors2);
// 匹配特征点
Ptr<DescriptorMatcher> matcher = DescriptorMatcher::create("BruteForce-Hamming");
vector<DMatch> matches;
matcher->match(descriptors1, descriptors2, matches);
// 筛选匹配点
vector<Point2f> points1, points2;
for (int i = 0; i < matches.size(); i++)
{
points1.push_back(keypoints1[matches[i].queryIdx].pt);
points2.push_back(keypoints2[matches[i].trainIdx].pt);
}
// 估计仿射变换矩阵
Mat H = Mat::zeros(2, 3, CV_64F);
double ransacReprojThreshold = 3;
H = estimateAffinePartial2D(points1, points2, noArray(), RANSAC, ransacReprojThreshold);
// 对图像2进行仿射变换
Mat result;
warpAffine(image2, result, H, image1.size());
// 显示图像对齐结果
namedWindow("Image1", WINDOW_NORMAL);
namedWindow("Image2", WINDOW_NORMAL);
namedWindow("Aligned Image2", WINDOW_NORMAL);
imshow("Image1", image1);
imshow("Image2", image2);
imshow("Aligned Image2", result);
waitKey();
return 0;
}
```
以上代码中,首先读入两张待对齐的图像,并使用ORB算法提取图像的特征点,并计算特征点的描述子。然后,使用BruteForce-Hamming算法进行特征点匹配,并筛选出匹配点。接着,使用RANSAC算法估计仿射变换矩阵,并将其应用到图像2上进行仿射变换,从而实现图像间的坐标对齐。最后,将三张图像显示在窗口中,以便观察对齐结果。
需要注意的是,上述代码中的特征点提取、描述子计算、特征点匹配和仿射变换矩阵估计都可以使用OpenCV库中的函数来实现,更加方便和高效。
阅读全文
相关推荐
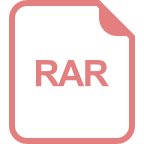
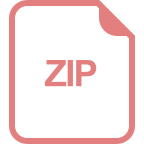
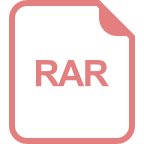
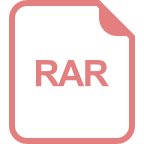
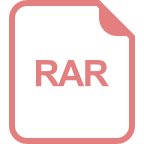
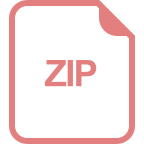
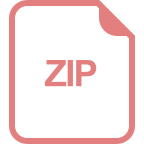
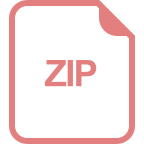
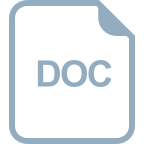
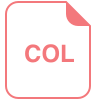
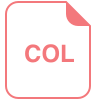
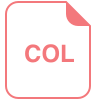
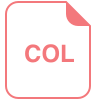
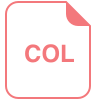
