2. Linux下,键盘输入文件名,父进程通过管道 发送该文件名给子进程,子进程获取文件名 后判断文件是否存在,之后,将文件是否存 在的信息通过管道告知父进程。
时间: 2024-02-27 08:58:37 浏览: 114
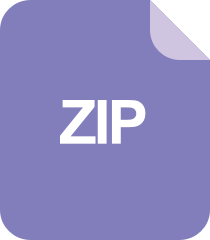
022批量获取指定文件夹下文件名并创建超链接共1页.pdf
好的,这是一个关于Linux下进程间通信的问题,我来为您解答。
首先,父进程需要创建一个管道,然后创建子进程。父进程从标准输入中获取文件名,将其写入管道中,子进程从管道中读取文件名,并在本地检查该文件是否存在。如果文件存在,子进程向管道中写入一个“1”,否则写入一个“0”。父进程从管道中读取这个信息,并进行相应的处理。
下面是一个示例代码,您可以参考一下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <sys/types.h>
#define BUFSIZE 512
int main() {
int fd[2];
pid_t pid;
char filename[BUFSIZE];
int file_exist = 0;
if (pipe(fd) < 0) {
perror("pipe error");
exit(1);
}
if ((pid = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid > 0) {
/* parent process */
close(fd[0]); // close read end of the pipe
printf("Enter filename: ");
scanf("%s", filename);
write(fd[1], filename, BUFSIZE); // write to the pipe
read(fd[0], &file_exist, sizeof(file_exist)); // read from the pipe
if (file_exist) {
printf("File exists\n");
} else {
printf("File does not exist\n");
}
close(fd[1]); // close write end of the pipe
} else {
/* child process */
close(fd[1]); // close write end of the pipe
read(fd[0], filename, BUFSIZE); // read from the pipe
if (access(filename, F_OK) != -1) {
file_exist = 1;
}
write(fd[1], &file_exist, sizeof(file_exist)); // write to the pipe
close(fd[0]); // close read end of the pipe
}
return 0;
}
```
在上面的代码中,父进程从标准输入中获取文件名,将其写入管道中;子进程从管道中读取文件名,并在本地检查该文件是否存在,将结果写入管道中。最后,父进程从管道中读取文件是否存在的信息,并进行相应的处理。
阅读全文
相关推荐
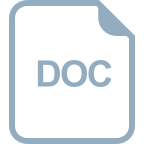
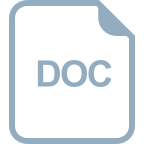

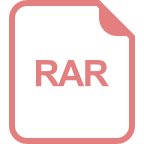
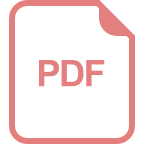
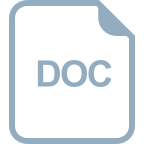
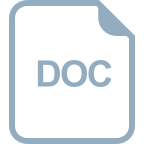
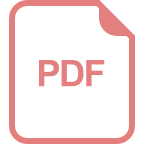
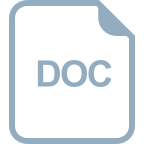
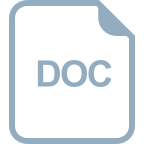
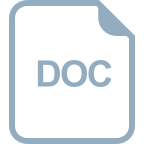
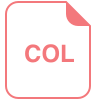
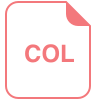
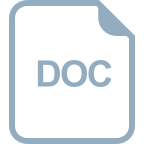
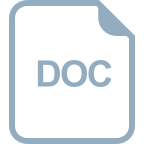