list按照年月分组,在年月分组的基础上,再用lambda表达式按照省份字段分组返回,Java开发中lambda如何写
时间: 2023-05-26 11:02:04 浏览: 72
假设有一个List,其中元素为如下格式的Map:
```java
Map<String, Object> item = new HashMap<>();
item.put("province", "广东");
item.put("year", 2021);
item.put("month", 8);
```
我们需要先按照年月进行分组,再按照省份进行分组。
```java
// 样例数据
List<Map<String, Object>> list = new ArrayList<>();
Map<String, Object> item1 = new HashMap<>();
item1.put("province", "广东");
item1.put("year", 2021);
item1.put("month", 8);
Map<String, Object> item2 = new HashMap<>();
item2.put("province", "广东");
item2.put("year", 2021);
item2.put("month", 7);
Map<String, Object> item3 = new HashMap<>();
item3.put("province", "广西");
item3.put("year", 2021);
item3.put("month", 8);
Map<String, Object> item4 = new HashMap<>();
item4.put("province", "广西");
item4.put("year", 2020);
item4.put("month", 8);
list.add(item1);
list.add(item2);
list.add(item3);
list.add(item4);
// 按照年月分组,在年月分组的基础上,再用lambda表达式按照省份字段分组返回
Map<Integer, Map<Integer, Map<String, List<Map<String, Object>>>>> result =
list.stream()
.collect(Collectors.groupingBy(
item -> (int) item.get("year"),
Collectors.groupingBy(
item -> (int) item.get("month"),
Collectors.groupingBy(
item -> (String) item.get("province"),
Collectors.toList()
)
)
));
```
上述lambda表达式中,我们使用了`Collectors.groupingBy()`方法进行分组。第一个参数表示按照哪个字段进行分组,第二个参数表示该组的元素如何处理,这里我们用了另一个`Collectors.groupingBy()`来进行嵌套分组,并在最里层使用了`Collectors.toList()`将该组下的元素放到一个List中。
结果的数据结构为:
```java
{
2020={
8={
广西=[
{province=广西, year=2020, month=8}
]
}
},
2021={
7={
广东=[
{province=广东, year=2021, month=7}
]
},
8={
广东=[
{province=广东, year=2021, month=8}
],
广西=[
{province=广西, year=2021, month=8}
]
}
}
}
```
相关推荐
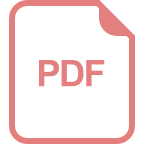
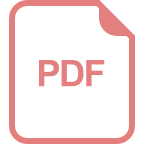
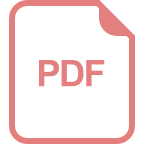
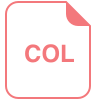
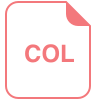
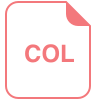
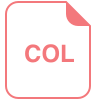
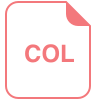









