close all; clear all; clc; load ('6mm_matlab.mat') % 相机标定基本参数 M = cameraParams.IntrinsicMatrix'; R = cameraParams.RotationMatrices(:,:,1); T = cameraParams.TranslationVectors(1,:)'; UV = cameraParams.ReprojectedPoints(:,:,1); % 将标定板角点像素坐标转换成齐次 for i = 1:size(UV,1) UV_H(i,:) = [UV(i,:),1]; end % 将像素坐标系转换到像素坐标系:参考https://blog.csdn.net/qq_43222384/article/details/101516807 leftMatrix = inv(R)*inv(M)*UV_H'; rightMatrix = inv(R)*T; rightMatrix_H = repmat(rightMatrix(3),[1,size(UV,1)]); temp_s = rightMatrix_H./leftMatrix(3,:); N_rightMatrix = repmat(rightMatrix,1,size(UV,1)); CB_World = temp_s.*leftMatrix - N_rightMatrix; WorldPoint = cameraParams.WorldPoints';
时间: 2023-12-06 22:40:05 浏览: 101
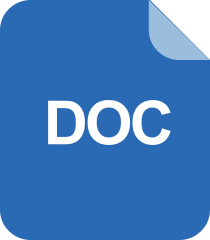
matlab 相机标定
这段代码是用于相机标定后计算标定板上角点的世界坐标的。具体来说,它假设已经知道了相机的内参矩阵、旋转矩阵和平移向量,以及标定板上角点在图像中的像素坐标,然后通过一系列的矩阵运算,计算出标定板上角点的世界坐标。
具体来说,代码中首先将相机内参矩阵和旋转矩阵求逆,并将像素坐标系下的角点像素坐标转换成齐次坐标。然后通过矩阵运算,将像素坐标系下的角点转换到相机坐标系下的坐标,并进一步转换到世界坐标系下的坐标。最后,将计算出的世界坐标保存在变量"WorldPoint"中。
阅读全文
相关推荐
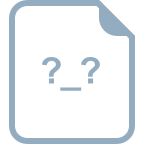










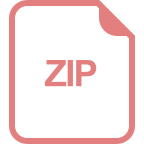
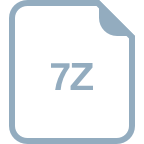
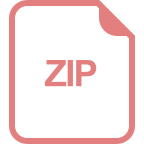
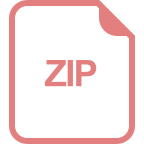
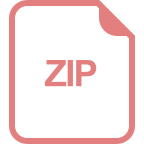
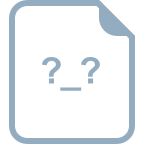