如何将测试集的正确标签和模型预测标签写入csv文档,python代码
时间: 2024-03-04 07:48:20 浏览: 15
下面是一个示例代码,可以将测试集的正确标签和模型预测标签写入一个csv文档中:
``` python
import pandas as pd
# 假设测试集的正确标签保存在test_labels列表中,模型预测标签保存在pred_labels列表中
test_labels = [0, 1, 0, 1, 1]
pred_labels = [1, 1, 0, 0, 1]
# 将标签数据转换为DataFrame格式
df = pd.DataFrame({'test_labels': test_labels, 'pred_labels': pred_labels})
# 将DataFrame保存为csv文件
df.to_csv('labels.csv', index=False)
```
在上面的示例代码中,首先将测试集的正确标签和模型预测标签保存在两个列表中,然后使用pandas库将这两个列表转换为DataFrame格式,并将其保存为名为"labels.csv"的csv文件。其中,`index=False`参数表示不保存行索引。你可以根据自己的需要修改代码中的变量名和文件名。
相关问题
python写入csv文档代码
写入CSV文档的代码如下:
```python
import csv
# 要写入的数据
data = [['name', 'age', 'gender'],
['Tom', '18', 'male'],
['Lucy', '20', 'female'],
['Jerry', '22', 'male']]
# 写入CSV文件
with open('data.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
for row in data:
writer.writerow(row)
```
在这个例子中,我们首先定义了一个二维列表data,其中包含了要写入的数据。
接着,我们使用Python内置的csv模块打开一个CSV文件,并创建一个csv.writer对象,用于将数据写入CSV文件中。
最后,我们通过循环遍历数据并调用writer.writerow()方法将每一行数据写入CSV文件中。
注意,在打开CSV文件时,我们要设置newline参数为'',以免写入的数据中出现额外的空行。
python补写写入csv文档代码
下面是一个简单的例子,将一些数据写入 CSV 文件中:
```python
import csv
# 数据
data = [
['Tom', 28],
['Jerry', 32],
['Spike', 25]
]
# 写入 CSV 文件
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
```
在这个例子中,我们使用了 CSV 模块中的 `csv.writer()` 函数创建了一个写入器对象,通过它可以将数据写入 CSV 文件中。
`csv.writer()` 函数接受一个文件对象以及一些可选参数,其中最重要的是 `newline` 参数,它用于指定行结束符。如果不指定该参数,写入的文件中可能会出现多余的空行。
`writerows()` 方法用于将多个行写入 CSV 文件,它接受一个可迭代对象,每个元素表示一行数据,每个元素又是一个列表,其中包含了每行数据的各个字段。
相关推荐
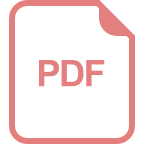
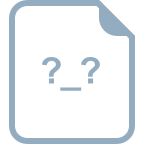












