with open('.\stop_words.txt', 'r', encoding='utf-8') as f: stop_words = f.read().split('\n')是什么意思
时间: 2023-08-11 07:03:43 浏览: 45
这段代码是用来读取名为 "stop_words.txt" 的文件中的内容,并将其存储到一个名为 stop_words 的列表中。具体来说,with open() 是 Python 中文件操作的一种简便方式,它可以在代码块执行完毕后自动关闭文件。其中,'\' 是用来转义的特殊字符,所以要在路径中使用两个反斜杠,即 '.\\stop_words.txt' 或者使用正斜杠,即 './stop_words.txt'。'r' 表示以只读方式打开文件,encoding='utf-8' 表示使用 utf-8 编码读取文件内容。read() 方法用于读取文件的全部内容,split('\n') 方法则用于将读取到的内容按照换行符 '\n' 进行分割,并将分割后的字符串存储到一个列表中。最终,这个列表中的每个元素都是 stop_words.txt 文件中的一行文本。
相关问题
encoding=utf-8 import nltk import json from nltk.corpus import stopwords import re eg_stop_words = set(stopwords.words('english')) sp_stop_words = set(stopwords.words('spanish')) all_stop_words = eg_stop_words.union(sp_stop_words) input_file_name = r'建模.txt' output_file_name = r'train.txt' out_file = open(output_file_name, encoding='utf-8', mode='w') 打开输出文件 with open(output_file_name, encoding='utf-8', mode='w') as output_file: # 打开输入文件,对每一行进行处理 with open(input_file_name, encoding='utf-8') as f: for idx, line in enumerate(f): print("正在处理第{}行数据".format(idx)) if idx == 0: # 第一行是列名, 不要 print(line) continue line = line.strip() sps = line.split("\t") # 将行按制表符分隔为列表 report_no = sps[0] target = sps[2] smses = sps[-1] smses = smses.strip(""") # 去掉短信两端的引号 smses = smses.replace("""", """) # 把两个双引号转换成单引号 root = json.loads(smses) # 解析 json 格式的短信 msg = "" for item in root: # 遍历短信中的每一条信息 body = item["body"] # 获取信息的正文 msg += body + "\n" # 把正文追加到总的信息传递过来的msg中 text = re.sub(r'[^\w\s]', '', msg) # 使用正则表达式去掉标点符号 text = re.sub(r'http\S+', '', text) # 去掉链接 text = re.sub(r'\d+', '', text)#去除数字 text = text.lower() words = text.split() filtered_words = [word for word in words if word not in all_stop_words] text = ' '.join(filtered_words) print(report_no + '\t' + target) msg = target + '\u0001' + text + '\n' out_file.write(msg) out_file.close()帮我改成用 pandas 处理
当使用 pandas 进行处理时,你可以使用 `pandas.read_csv()` 函数读取文件,并使用 DataFrame 进行数据处理。以下是使用 pandas 进行处理的示例代码:
```python
import pandas as pd
import nltk
from nltk.corpus import stopwords
import re
eg_stop_words = set(stopwords.words('english'))
sp_stop_words = set(stopwords.words('spanish'))
all_stop_words = eg_stop_words.union(sp_stop_words)
input_file_name = '建模.txt'
output_file_name = 'train.txt'
df = pd.read_csv(input_file_name, encoding='utf-8', sep='\t')
# 处理每一行数据
for idx, row in df.iterrows():
print("正在处理第{}行数据".format(idx))
if idx == 0:
# 第一行是列名,不需要处理
continue
smses = row['短信']
smses = smses.strip("'")
smses = smses.replace('""', "'")
root = json.loads(smses)
msg = ""
for item in root:
body = item["body"]
msg += body + "\n"
text = re.sub(r'[^\w\s]', '', msg)
text = re.sub(r'http\S+', '', text)
text = re.sub(r'\d+', '', text)
text = text.lower()
words = text.split()
filtered_words = [word for word in words if word not in all_stop_words]
text = ' '.join(filtered_words)
df.at[idx, '目标'] = row['目标'] + '\u0001' + text
df.to_csv(output_file_name, encoding='utf-8', sep='\t', index=False)
```
这段代码将会读取名为 '建模.txt' 的文件,并根据每一行的数据进行处理,最后将处理后的结果保存到 'train.txt' 文件中。注意在使用该代码前,你需要先安装 pandas 和 nltk 库。
import pandas as pd import jieba from wordcloud import WordCloud import matplotlib.pyplot as plt from PIL import Image # 读取中间表数据并提取读者ID和图书ID列 df = pd.read_excel('中间表.xlsx') reader_ids = df['读者ID'] book_ids = df['图书ID'] # 根据读者ID和图书ID关联读者信息和图书目录,得到每个读者借阅的图书的书名 readers_info = pd.read_excel('读者信息.xlsx') books_catalog = pd.read_excel('图书目录.xlsx') books_borrowed = books_catalog[books_catalog['图书ID'].isin(book_ids)] borrowed_books_names = books_borrowed['书名'] # 使用jieba进行中文分词 split_words = [] for book_name in borrowed_books_names: words = jieba.lcut(book_name) split_words.extend(words) # 加载停用词表并进行停用词过滤 stop_words_files = ['停用词表1.txt', '停用词表2.txt', '停用词表3.txt'] stop_words = set() for stop_words_file in stop_words_files: with open(stop_words_file, 'r', encoding='utf-8') as f: stop_words |= set(f.read().splitlines()) filtered_words = [word for word in split_words if word not in stop_words] # 加载篮球形状图片并生成词云图 basketball_mask = np.array(Image.open('basketball.png')) wordcloud = WordCloud(font_path='simhei.ttf', background_color='white', mask=basketball_mask).generate(' '.join(filtered_words)) plt.imshow(wordcloud, interpolation='bilinear') plt.axis('off') plt.show() # 获取词频最高的前10个词语 word_counts = pd.Series(filtered_words).value_counts() top_10_words = word_counts.head(10).index.tolist() print("该专业师生最迫切需要学习的知识:", top_10_words)
这段代码的作用是生成一个词云图,并输出该专业师生最迫切需要学习的知识(词频最高的前10个词语)。代码中使用了pandas库来读取和处理Excel文件数据,jieba库进行中文分词,wordcloud库生成词云图,matplotlib库进行图像展示,PIL库进行图片处理。
在代码中,使用`PIL.Image.open()`函数加载了一张名为'basketball.png'的图片作为词云图的形状模板。请确保'basketball.png'文件存在,并且与代码文件在同一目录下。
此外,代码还使用了一些Excel文件('中间表.xlsx'、'读者信息.xlsx'、'图书目录.xlsx'),请确保这些文件存在并包含正确的数据。
停用词表文件('停用词表1.txt'、'停用词表2.txt'、'停用词表3.txt')应该是包含一些常用词语的文本文件,每行一个词语。请确保这些文件存在,并且以UTF-8编码保存。
最后,代码输出了词频最高的前10个词语。请注意,此处涉及到`simhei.ttf`字体文件,确保该字体文件存在并与代码文件在同一目录下。
如果您遇到了任何错误,请提供具体的错误信息以便我更准确地帮助您解决问题。
相关推荐
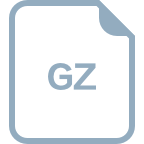
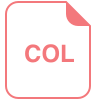
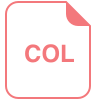
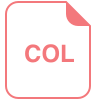
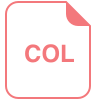
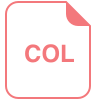








