编写个罗盘时钟代码 实现可以在电脑桌面直接展示
时间: 2024-02-27 14:51:39 浏览: 127
以下是使用Python和Tkinter库编写的罗盘时钟代码,可以在电脑桌面直接展示:
```python
import tkinter as tk
import math
import time
class CompassClock:
def __init__(self, root):
self.root = root
self.root.resizable(width=False, height=False)
self.root.attributes('-topmost', True)
self.root.overrideredirect(True)
self.width = 200
self.height = 200
self.canvas = tk.Canvas(self.root, width=self.width, height=self.height)
self.canvas.pack()
self.draw_compass()
self.update_clock()
def draw_compass(self):
cx = self.width // 2
cy = self.height // 2
r = min(cx, cy) - 10
self.canvas.create_oval(cx-r, cy-r, cx+r, cy+r, width=2)
for i in range(0, 360, 30):
x1 = cx + r * math.sin(math.radians(i))
y1 = cy - r * math.cos(math.radians(i))
x2 = cx + (r-10) * math.sin(math.radians(i))
y2 = cy - (r-10) * math.cos(math.radians(i))
self.canvas.create_line(x1, y1, x2, y2, width=2)
self.hour_hand = self.canvas.create_line(cx, cy, cx, cy-r//2, width=3, fill='red')
self.minute_hand = self.canvas.create_line(cx, cy, cx, cy-r*3//4, width=2, fill='blue')
self.second_hand = self.canvas.create_line(cx, cy, cx, cy-r*3//4, width=1, fill='green')
def update_clock(self):
current_time = time.localtime()
hour = current_time.tm_hour % 12
minute = current_time.tm_min
second = current_time.tm_sec
hour_angle = (hour * 30) + (minute / 2)
minute_angle = minute * 6
second_angle = second * 6
self.canvas.delete(self.hour_hand)
self.canvas.delete(self.minute_hand)
self.canvas.delete(self.second_hand)
cx = self.width // 2
cy = self.height // 2
r = min(cx, cy) - 10
x1 = cx + (r-20) * math.sin(math.radians(hour_angle))
y1 = cy - (r-20) * math.cos(math.radians(hour_angle))
self.hour_hand = self.canvas.create_line(cx, cy, x1, y1, width=3, fill='red')
x2 = cx + (r-10) * math.sin(math.radians(minute_angle))
y2 = cy - (r-10) * math.cos(math.radians(minute_angle))
self.minute_hand = self.canvas.create_line(cx, cy, x2, y2, width=2, fill='blue')
x3 = cx + r * math.sin(math.radians(second_angle))
y3 = cy - r * math.cos(math.radians(second_angle))
self.second_hand = self.canvas.create_line(cx, cy, x3, y3, width=1, fill='green')
self.root.after(1000, self.update_clock)
if __name__ == '__main__':
root = tk.Tk()
clock = CompassClock(root)
root.mainloop()
```
将此代码保存为.py文件,然后运行即可在电脑桌面上展示罗盘时钟。
阅读全文
相关推荐

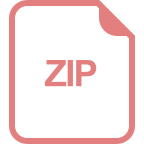
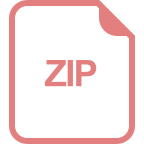
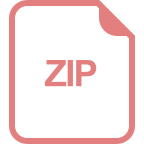
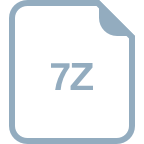
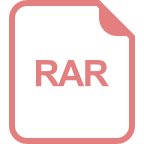
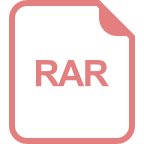
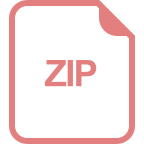
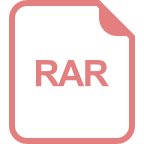
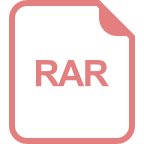
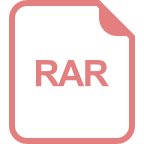
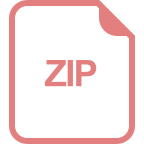
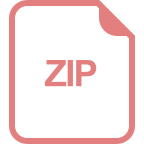
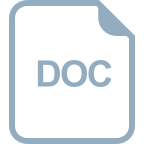
