python 让可视化窗口不消失
时间: 2023-06-26 16:03:32 浏览: 109
在Python中,如果你使用了一些可视化库(如Matplotlib等),程序运行后,窗口会自动消失。这是因为程序执行完毕后,窗口就会关闭。
如果你想让窗口保持打开状态,可以使用以下两种方法:
1. 在程序最后加上`plt.show()`语句,这样程序会一直运行,直到你手动关闭窗口。
```python
import matplotlib.pyplot as plt
# 绘制图形
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('My Plot')
# 显示图形
plt.show()
```
2. 使用`plt.ion()`函数,在程序执行时开启交互模式,这样窗口就不会自动关闭了。
```python
import matplotlib.pyplot as plt
# 开启交互模式
plt.ion()
# 绘制图形
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('My Plot')
# 显示图形
plt.show()
# 程序继续执行,窗口不会自动关闭
input('Press enter to continue...')
# 关闭交互模式
plt.ioff()
```
相关问题
python怎么创建可视化窗口
要在 Python 中创建可视化窗口,您可以使用许多不同的 GUI 工具包,包括 Tkinter、PyQt、PySide、wxPython 等等。其中,Tkinter 是 Python 内置的 GUI 工具包,使用起来比较简单,适合初学者。
下面是一个使用 Tkinter 创建一个简单窗口的示例代码:
```python
import tkinter as tk
# 创建窗口对象
window = tk.Tk()
# 设置窗口标题
window.title("Hello, World!")
# 设置窗口大小
window.geometry("300x200")
# 显示窗口
window.mainloop()
```
这个示例代码创建了一个窗口对象,设置了窗口标题和大小,并通过 `mainloop()` 方法显示窗口。
您可以根据自己的需求添加更多的 GUI 组件,例如标签、按钮、文本框等等。Tkinter 提供了大量的 GUI 组件,详细使用方法可以参考官方文档。
python怎么做可视化窗口界面
Python有多种可视化窗口界面的库可以选择,其中比较常用的是Tkinter、PyQt、wxPython和PyGTK等。下面以Tkinter为例,介绍一下如何制作简单的窗口界面。
1.导入Tkinter库
```python
import tkinter as tk
```
2.创建窗口
```python
window = tk.Tk() #创建主窗口
window.title("窗口标题") #设置窗口标题
window.geometry("400x300") #设置窗口大小
```
3.添加控件
```python
label = tk.Label(window, text="标签") #创建标签控件
label.pack() #将控件添加到窗口中
button = tk.Button(window, text="按钮") #创建按钮控件
button.pack() #将控件添加到窗口中
```
4.事件处理
```python
def button_click():
print("按钮被点击了")
button = tk.Button(window, text="按钮", command=button_click) #将按钮点击事件与函数绑定
```
5.启动窗口事件循环
```python
window.mainloop()
```
以上是简单的窗口界面创建过程,你可以根据需要添加更多的控件和事件处理。
相关推荐
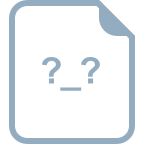
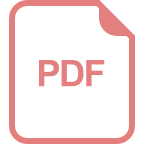












