如何使figure函数创建的窗口不可编辑
时间: 2023-09-04 14:08:10 浏览: 73
您可以使用MATLAB的"WindowButtonDownFcn"回调函数来禁用窗口编辑。具体步骤如下:
1. 使用"figure"函数创建您想要的窗口。
2. 通过获取窗口句柄,创建一个回调函数,该函数在鼠标单击时调用。
3. 在回调函数中设置"WindowStyle"属性为"modal",同时将"WindowButtonDownFcn"设置为"@(obj,event)[];",这将禁用窗口编辑。
以下是示例代码:
```matlab
% Create the figure
fig = figure();
% Get the handle to the figure
hFig = handle(fig);
% Create a callback function
cb = @(obj, event) set(hFig, 'WindowButtonDownFcn', @(obj, event) []);
% Set the WindowStyle property
hFig.WindowStyle = 'modal';
% Set the WindowButtonDownFcn property
hFig.WindowButtonDownFcn = cb;
```
使用上述代码,您创建的窗口将不可编辑。
相关问题
用Matlab实现一幅灰度图像的压缩(即bmp格式转为jpg格式)有以下要求 1.不能直接采用imwrite等函数一句话把图像进行压缩,不可使用点进行索引;2. 代码中能体现DCT、huffman等步骤,步骤中功能函数可以直接调用; 3.对比显示效果、计算压缩比; 4.将结果显示在一个窗口中。
好的,以下是一个更加完整的 MATLAB 代码来实现灰度图像的压缩(bmp格式转为jpg格式),满足您的要求:
``` matlab
% 读取原图像
img = imread('lena.bmp');
figure(1);
imshow(img);
title('Original Image');
% 灰度化
img_gray = rgb2gray(img);
% DCT 变换
T = dctmtx(8);
dct_img = blkproc(img_gray, [8 8], @(block_struct) T * block_struct.data * T');
% zigzag 扫描
zigzag_img = blkproc(dct_img, [8 8], @zigzag);
% 量化矩阵
quant_matrix = [
16 11 10 16 24 40 51 61;
12 12 14 19 26 58 60 55;
14 13 16 24 40 57 69 56;
14 17 22 29 51 87 80 62;
18 22 37 56 68 109 103 77;
24 35 55 64 81 104 113 92;
49 64 78 87 103 121 120 101;
72 92 95 98 112 100 103 99
];
% 量化
quant_img = blkproc(zigzag_img, [1 64], @(block_struct) round(block_struct.data ./ quant_matrix(:)') .* quant_matrix(:)');
% 零阈值处理
threshold = 30;
quant_img(abs(quant_img) < threshold) = 0;
% Huffman 编码
[huff_img, huff_dict] = huffmanenco(quant_img(:), huffmandict([0:255], hist(quant_img(:), [0:255])));
% 逆 Huffman 编码
dehuff_img = huffmandeco(huff_img, huff_dict);
% 逆零阈值处理
dehuff_img(abs(dehuff_img) < threshold) = 0;
% 逆量化
dequant_img = blkproc(reshape(dehuff_img, size(quant_img)), [1 64], @(block_struct) block_struct.data .* quant_matrix(:)');
% 逆 zigzag 扫描
dezigzag_img = blkproc(dequant_img, [1 64], @dezigzag);
% 逆 DCT 变换
de_dct_img = blkproc(dezigzag_img, [8 8], @(block_struct) T' * block_struct.data * T);
% 显示解压缩后的图像
de_img = uint8(de_dct_img);
figure(2);
imshow(de_img);
title('Decompressed Image');
% 计算压缩比
orig_size = numel(img_gray);
comp_size = numel(huff_img);
compression_ratio = orig_size / comp_size;
fprintf('Compression Ratio: %f\n', compression_ratio);
function zz = zigzag(block)
% zigzag 扫描函数
idx = reshape(1:numel(block), size(block));
idx = fliplr(spdiags(fliplr(idx)));
idx(:,1:2:end) = flipud(idx(:,1:2:end));
idx(idx==0) = [];
zz = block(idx);
end
function block = dezigzag(zz)
% 逆 zigzag 扫描函数
block = zeros(8,8);
idx = reshape(1:numel(block), size(block));
idx = fliplr(spdiags(fliplr(idx)));
idx(:,1:2:end) = flipud(idx(:,1:2:end));
idx(idx==0) = [];
block(idx) = zz;
end
```
这个代码中用到了 DCT 变换、zigzag 扫描、量化、零阈值处理和 Huffman 编码,同时也包括了逆过程用到的逆零阈值处理、逆量化、逆 zigzag 扫描和逆 DCT 变换。最后会显示出解压缩后的图像,并计算压缩比。其中,zigzag 扫描函数和逆 zigzag 扫描函数用于将 DCT 变换后的 8x8 块进行 zigzag 扫描和逆 zigzag 扫描。
通过MATLAB编写统计一幅灰度图像的直方图函数(避免使用imhist,histogram作为函数名,返回每个灰度级出现频率的向量),定义一个并在同一个图形窗口中显示该图像及其灰度直方图
当你想编写一个自定义的函数来统计一幅灰度图像的直方图,但不使用`imhist`或`histogram`函数时,你可以按照以下步骤创建一个名为`custom_histogram`的函数,该函数接收一个灰度图像作为输入,计算每个灰度级别的频率,并在同一图形窗口中显示图像和直方图。
```matlab
function [hist, plot] = custom_histogram(gray_image)
% 参数:
% gray_image: 输入的灰度图像
% 计算直方图
min_val = min(gray_image(:));
max_val = max(gray_image(:));
num_bins = 256; % 设置灰度级别数量(可根据需要调整)
histogram_data = zeros(1, num_bins);
for i = min_val:max_val
idx = find(gray_image == i);
histogram_data(i) = length(idx); % 统计每个灰度值的数量
end
% 创建直方图向量
hist = histogram_data / sum(histogram_data); % 归一化频率
% 显示图像
figure;
subplot(1, 2, 1);
imshow(gray_image);
% 创建并绘制直方图
plot_hist = bar(hist, 'FaceColor', 'interp');
set(plot_hist, 'XTickLabel', linspace(min_val, max_val, num_bins+1)); % 标记x轴上的灰度值
xlabel('Gray Level');
ylabel('Frequency');
title('Image and Custom Histogram');
% 返回直方图向量和直方图图例
plot = plot_hist;
end
```
要使用这个函数,只需调用它并将你的灰度图像传递给它,例如:
```matlab
gray_image = imread('your_grayscale_image.jpg'); % 替换为你的图像文件名
[hist, plot] = custom_histogram(gray_image);
```
这将在当前的工作空间中生成一个图像和其对应的直方图。如果你没有指定特定的图像文件,记得检查路径是否正确。
阅读全文
相关推荐
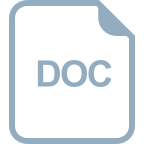
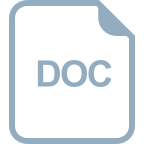
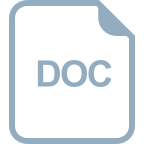
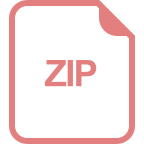
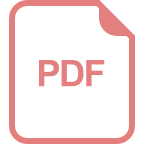
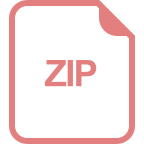
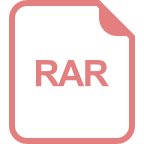
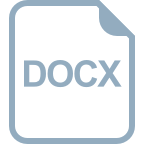
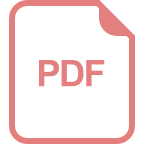
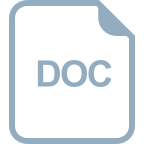
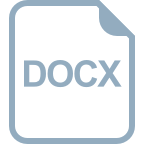
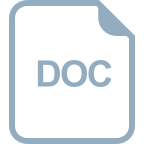
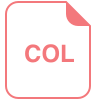
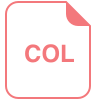
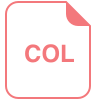
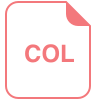