用c语言实现以下功能:设计单链表,用于存储地址、单号、姓名、电话信息,并将录入的信息按地址为主关键字、单号为次关键字进行排序,最后输入姓名电话信息能查询到单号信息
时间: 2023-08-16 17:09:39 浏览: 69
好的,我会尽力回答您的问题。
以下是用C语言实现的单链表,用于存储地址、单号、姓名、电话信息,并按地址为主关键字、单号为次关键字进行排序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node
{
char address[20];
int number;
char name[20];
char phone[12];
struct node *next;
} Node;
Node *create_list();
void print_list(Node *head);
void sort_list(Node *head);
void search_list(Node *head);
int main()
{
Node *head = create_list();
sort_list(head);
print_list(head);
search_list(head);
return 0;
}
Node *create_list()
{
Node *head = NULL;
Node *tail = NULL;
char address[20];
int number;
char name[20];
char phone[12];
printf("Enter address, number, name, and phone number (separated by spaces, end with -1):\n");
scanf("%s %d %s %s", address, &number, name, phone);
while (number != -1)
{
Node *new_node = (Node *)malloc(sizeof(Node));
strcpy(new_node->address, address);
new_node->number = number;
strcpy(new_node->name, name);
strcpy(new_node->phone, phone);
new_node->next = NULL;
if (head == NULL)
{
head = new_node;
tail = new_node;
}
else
{
tail->next = new_node;
tail = new_node;
}
scanf("%s %d %s %s", address, &number, name, phone);
}
return head;
}
void print_list(Node *head)
{
printf("Address\tNumber\tName\tPhone\n");
printf("----------------------------------------\n");
Node *p = head;
while (p != NULL)
{
printf("%s\t%d\t%s\t%s\n", p->address, p->number, p->name, p->phone);
p = p->next;
}
printf("----------------------------------------\n");
}
void sort_list(Node *head)
{
Node *p = head;
Node *q = NULL;
int temp_number;
char temp_address[20], temp_name[20], temp_phone[12];
while (p != NULL)
{
q = p->next;
while (q != NULL)
{
if (strcmp(p->address, q->address) > 0)
{
temp_number = p->number;
strcpy(temp_address, p->address);
strcpy(temp_name, p->name);
strcpy(temp_phone, p->phone);
p->number = q->number;
strcpy(p->address, q->address);
strcpy(p->name, q->name);
strcpy(p->phone, q->phone);
q->number = temp_number;
strcpy(q->address, temp_address);
strcpy(q->name, temp_name);
strcpy(q->phone, temp_phone);
}
else if (strcmp(p->address, q->address) == 0 && p->number > q->number)
{
temp_number = p->number;
strcpy(temp_address, p->address);
strcpy(temp_name, p->name);
strcpy(temp_phone, p->phone);
p->number = q->number;
strcpy(p->address, q->address);
strcpy(p->name, q->name);
strcpy(p->phone, q->phone);
q->number = temp_number;
strcpy(q->address, temp_address);
strcpy(q->name, temp_name);
strcpy(q->phone, temp_phone);
}
q = q->next;
}
p = p->next;
}
}
void search_list(Node *head)
{
char name[20];
printf("Please enter the name you want to search: ");
scanf("%s", name);
Node *p = head;
while (p != NULL)
{
if (strcmp(p->name, name) == 0)
{
printf("%s's number is %d\n", p->name, p->number);
return;
}
p = p->next;
}
printf("Sorry, we could not find the information for %s\n", name);
}
```
在这个代码中,我们定义了一个 `Node` 结构体,包含了地址、单号、姓名和电话信息,以及指向下一个节点的指针。然后我们通过 `create_list()` 函数创建了一个单链表,并通过键盘输入录入了信息。接着我们通过 `sort_list()` 函数对链表进行了排序,按照地址为主关键字,单号为次关键字。最后通过 `print_list()` 函数输出了排序后的链表,并通过 `search_list()` 函数实现了通过姓名查询单号信息的功能。
希望这个代码对您有所帮助!
相关推荐
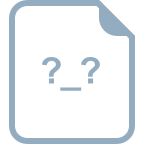
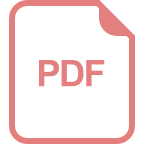
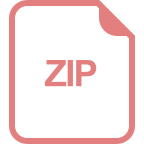














