python实现从pdf提取文字,图片,表格和标题
时间: 2024-10-17 22:05:00 浏览: 159
在Python中,可以使用一些专门库来从PDF文件中提取文本、图片、表格和标题等信息。以下是几个常用的工具:
1. **Text Extraction**:
- `PyPDF2`: 这是一个基本的PDF读取库,用于提取文本内容。例如:
```python
import PyPDF2
with open('example.pdf', 'rb') as file:
pdf = PyPDF2.PdfFileReader(file)
for page_num in range(pdf.getNumPages()):
text = pdf.getPage(page_num).extractText()
print(text)
```
2. **Image Extraction**:
- `PIL (Pillow)`: 可以用于处理图像数据,包括PDF中的图片。首先,需要将PDF转换为包含嵌入图片的HTML或XML格式,然后解析出来:
```python
from pdf2image import convert_from_path
images = convert_from_path('example.pdf')
for i, img in enumerate(images):
img.save(f"image_{i}.png")
```
3. **Table Extraction**:
- `tabula-py` 和 `camelot`: 这些库适用于识别并提取PDF中的表格,通常适用于结构化的数据。
```python
import tabula
df = tabula.read_pdf('example.pdf', pages='all')
# 或者对于Camelot:
from camelot import read_pdf
tables = read_pdf('example.pdf', flavor='stream')
```
4. **Title Extraction**:
- 对于PDF文档,标题通常出现在元数据或页面元素中。你可以通过`PyPDF2`查找特定词汇或者使用`PDFMiner`来提取文档标题。
```python
from pdfminer.high_level import extract_text_to_string
with open('example.pdf', 'rb') as file:
metadata = PDFMiner.get_document_info(file)
title = metadata.get("/Title", "No Title Found")
print(title)
```
请注意,实际操作可能因PDF的内容复杂性和格式的不同而有所差异。
阅读全文
相关推荐
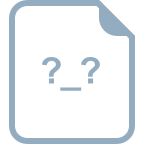
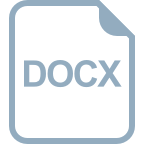
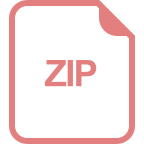
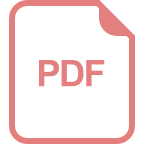
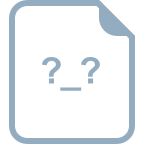
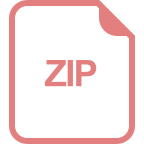
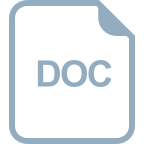
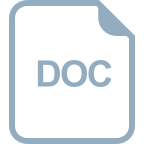







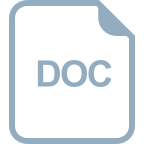

