图书链表操作实现的代码
时间: 2024-10-14 09:09:07 浏览: 18
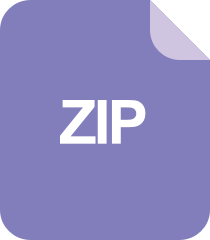
代码_反转链表_
图书链表通常是一种数据结构,用于存储书籍信息,每个节点代表一本书,包含书名、作者等字段,并链接到下一个节点。以下是使用Python语言简单地描述一个图书链表的基本操作实现:
```python
class BookNode:
def __init__(self, title, author):
self.title = title
self.author = author
self.next = None
class LibraryList:
def __init__(self):
self.head = None
# 插入一本书
def add_book(self, title, author):
new_node = BookNode(title, author)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
# 查找特定书名的书籍
def find_book(self, title):
if not self.head:
return None
current = self.head
while current:
if current.title == title:
return current
current = current.next
return None
# 删除一本书
def remove_book(self, title):
if not self.head:
return
if self.head.title == title:
self.head = self.head.next
return
current = self.head
prev = None
while current and current.title != title:
prev = current
current = current.next
if current is not None:
prev.next = current.next
# 示例操作
library_list = LibraryList()
library_list.add_book("图书A", "作者A")
library_list.add_book("图书B", "作者B")
# 查找并打印书籍
book = library_list.find_book("图书A")
if book:
print(f"找到书籍:{book.title} - {book.author}")
# 删除书籍
library_list.remove_book("图书B")
```
阅读全文
相关推荐
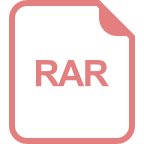
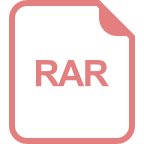
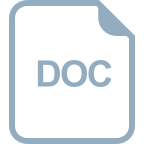
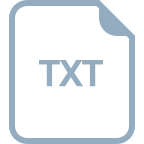
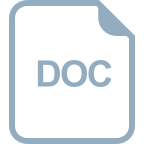
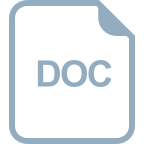
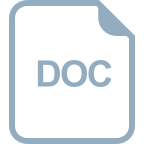
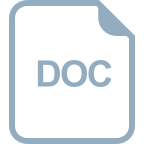
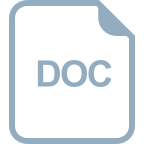
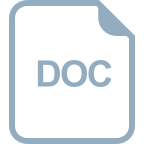
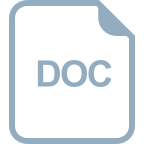
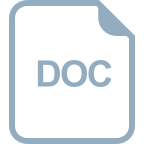
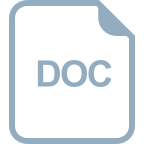
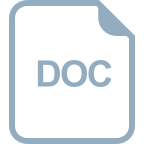
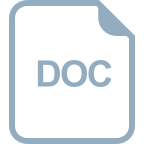
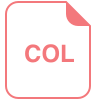
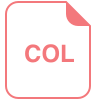
