python爬虫封装头部
时间: 2023-07-12 18:05:42 浏览: 159
在 Python 中,我们可以使用 requests 库来发送 HTTP 请求。要封装请求头,我们可以使用 requests 库的 headers 参数。下面是一个示例代码,演示如何在 Python 爬虫中封装请求头:
```python
import requests
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36 Edge/16.16299',
'Referer': 'https://www.baidu.com/',
'Accept-Encoding': 'gzip, deflate, br',
'Accept-Language': 'zh-CN,zh;q=0.9,en-US;q=0.8,en;q=0.7'
}
# 发送 HTTP 请求,并设置请求头
response = requests.get('https://www.example.com', headers=headers)
# 打印响应内容
print(response.text)
```
在这个例子中,我们使用了 requests 库的 get 方法发送了一个 HTTP GET 请求,并通过 headers 参数封装了请求头。请注意,headers 参数是一个字典,其中键是请求头的名称,值是请求头的值。
相关问题
帮我写一个Python爬虫框架
创建一个简单的Python爬虫框架,我们可以使用`requests`和`BeautifulSoup`这两个库。下面将指导您构建这样一个基本框架。
### 第一步:安装必要的库
首先,你需要在你的环境中安装`requests` 和 `beautifulsoup4`。你可以使用pip命令安装它们:
```bash
pip install requests beautifulsoup4
```
### 第二步:设计框架结构
我们定义一个基础的爬虫类,这个类会封装请求网页、解析HTML以及存储数据的功能。
#### 类结构说明:
1. **初始化方法** (`__init__`):设置默认参数,比如超时时间、请求头部信息等。
2. **获取网页源码** (`get_html(url)`):发送HTTP GET请求并返回响应的内容。
3. **解析HTML** (`parse_html(html_content)`):使用BeautifulSoup解析HTML内容,提取有用的数据。
4. **存储数据** (`store_data(data)`):根据需求将数据保存到文件或其他数据库。
### 实现代码
```python
import requests
from bs4 import BeautifulSoup
class SimpleSpider:
def __init__(self, timeout=5, headers={'User-Agent': 'Mozilla/5.0'}):
self.timeout = timeout
self.headers = headers
def get_html(self, url):
try:
response = requests.get(url, headers=self.headers, timeout=self.timeout)
if response.status_code == 200:
return response.text
else:
print(f"Failed to get the HTML content with status code {response.status_code}")
return None
except Exception as e:
print(f"Error occurred while getting the HTML content: {e}")
return None
def parse_html(self, html_content):
soup = BeautifulSoup(html_content, "html.parser")
# 这里假设页面有一个特定的标签用于抓取数据,例如所有链接
links = [a['href'] for a in soup.find_all('a')]
return links
def store_data(self, data):
filename = "data.txt"
with open(filename, 'w') as file:
for item in data:
file.write("%s\n" % item)
# 使用示例
spider = SimpleSpider()
url = "https://example.com" # 目标网站URL
content = spider.get_html(url)
if content is not None:
parsed_data = spider.parse_html(content)
spider.store_data(parsed_data)
else:
print("Could not fetch and process the HTML content.")
```
### 注意事项:
- 确保遵守目标网站的`robots.txt`规则和版权法律。
- 对于更复杂的爬虫,你可能还需要处理JavaScript渲染的页面、登录认证等问题。
- 考虑使用异步请求来提高速度,可以使用`asyncio`和`aiohttp`库。
以上就是一个基础的Python爬虫框架的实现,您可以根据实际需求调整和扩展功能。
阅读全文
相关推荐
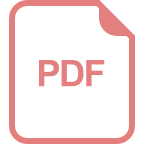
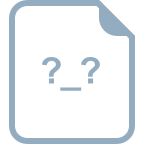
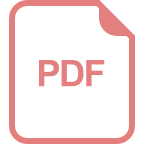
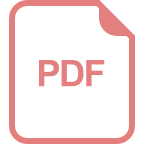
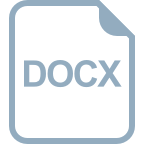
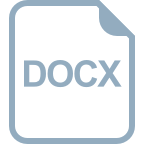
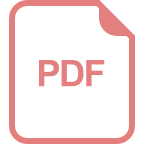
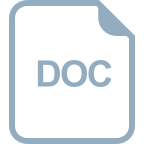
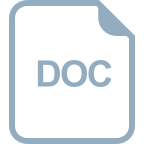
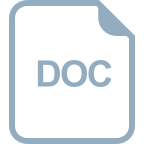
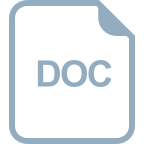
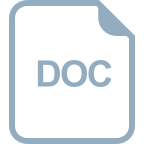
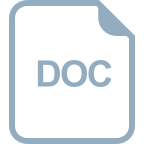
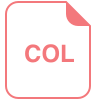
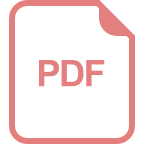
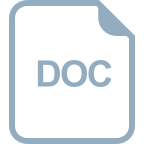
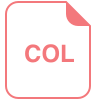