std::string <==> ::std::string
时间: 2023-11-21 17:05:28 浏览: 100
`std::string`和`::std::string`是完全相同的,它们都是C++标准库中的字符串类。`std`是C++标准库的命名空间,因此`std::string`表示在`std`命名空间中定义的字符串类。而`::std::string`中的`::`表示全局命名空间,因此它也表示相同的字符串类。两者可以互换使用,没有任何区别。
示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string str1 = "hello";
::std::string str2 = "world";
std::string str3 = str1 + ", " + str2;
std::cout << str3 << std::endl; // 输出:hello, world
return 0;
}
```
相关问题
std::string转vector<byte8>
在C++中,将`std::string`转换为`std::vector<uint8_t>`(通常称为byte数组)可以使用标准库提供的函数。首先,你需要包含必要的头文件:
```cpp
#include <string>
#include <vector>
#include <codecvt> // 如果你的编译器支持宽字符
// 使用std::wstring_convert将字符串转换为宽字符序列,然后转为字节
std::wstring_convert<std::codecvt_utf8<wchar_t>> converter;
std::wstring wstr = converter.from_bytes(your_string); // 将std::string转换为宽字符串
std::vector<uint8_t> byte_vector(wstr.begin(), wstr.end()); // 创建byte vector,存储宽字符串的内容
```
如果你处理的是ASCII字符,可以直接复制字符串内容到字节数组,因为它们的编码是一对一的关系:
```cpp
std::vector<uint8_t> byte_vector(your_string.begin(), your_string.end());
```
std::vector<std::string>
std::vector<std::string>是C++标准库中的一个容器类,用于存储一组字符串。它提供了动态数组的功能,可以根据需要自动调整大小。
使用std::vector<std::string>可以方便地进行字符串的添加、删除、查找等操作。以下是std::vector<std::string>的一些常用操作:
1. 添加元素:可以使用push_back()函数将一个字符串添加到std::vector<std::string>的末尾。
2. 删除元素:可以使用erase()函数删除指定位置的字符串,或者使用pop_back()函数删除末尾的字符串。
3. 访问元素:可以使用下标运算符[]来访问指定位置的字符串,也可以使用at()函数来进行访问,at()函数会进行边界检查。
4. 获取大小:可以使用size()函数获取std::vector<std::string>中元素的个数。
5. 清空容器:可以使用clear()函数清空std::vector<std::string>中的所有元素。
6. 遍历容器:可以使用迭代器来遍历std::vector<std::string>中的所有元素。
阅读全文
相关推荐
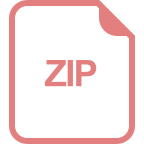
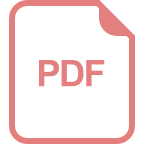
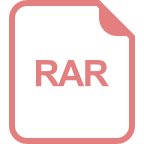













