std::map<std::string, std::string> dict
时间: 2024-05-27 22:07:07 浏览: 133
`std::map`是C++ STL中的一个关联容器,它提供了一种以“key-value”(键-值)形式存储数据的方式。对于`std::map<std::string, std::string>`类型的变量`dict`,它可以存储一组字符串-字符串的键值对。下面是一些`std::map`的简单操作演示:
1. 声明和初始化一个空的map对象
```c++
#include <map>
#include <iostream>
#include <string>
std::map<std::string, std::string> dict; // 声明一个空的map变量
```
2. 插入键值对到map中
```c++
dict.insert(std::pair<std::string, std::string>("apple", "a kind of fruit")); // 插入一组键值对
dict["banana"] = "another kind of fruit"; // 通过下标操作符插入一组键值对
```
3. 查找map中的键是否存在
```c++
if (dict.find("orange") == dict.end()) {
std::cout << "Key 'orange' not found in dict." << std::endl;
}
```
4. 删除map中的键值对
```c++
dict.erase("banana"); // 删除键为"banana"的键值对
```
5. 遍历map中的所有键值对
```c++
for (auto it = dict.begin(); it != dict.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
```
以上是一些基本的`std::map`操作,你也可以通过其他的STL算法来操作map。如果你需要更多关于`std::map`的知识,可以查看[C++ Reference](https://en.cppreference.com/w/cpp/container/map)。
阅读全文
相关推荐
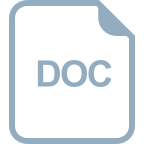
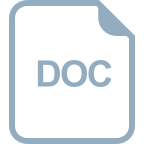
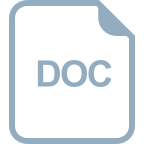
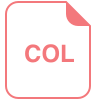


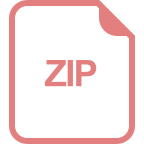
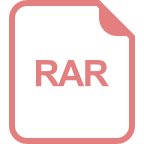
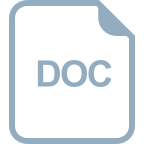
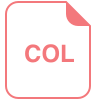








