java FTPFile文件下载
时间: 2024-05-09 13:12:09 浏览: 8
Java中的FTPFile类是用于表示远程FTP服务器上的文件或目录的类,可以通过FTP客户端连接到FTP服务器,并使用FTPClient类来下载文件。
以下是一个简单的示例代码,演示如何使用FTPClient和FTPFile来下载文件:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class FTPFileDownload {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String username = "username";
String password = "password";
String remoteFilePath = "/path/to/remote/file.txt";
String localFilePath = "/path/to/local/file.txt";
FTPClient ftpClient = new FTPClient();
try {
// 连接FTP服务器
ftpClient.connect(server, port);
ftpClient.login(username, password);
// 切换到二进制文件传输模式
ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE);
// 获取远程文件
FTPFile[] files = ftpClient.listFiles(remoteFilePath);
if (files.length != 1) {
throw new IOException("远程文件不存在或者有多个文件匹配:" + remoteFilePath);
}
FTPFile file = files;
// 创建输出流
OutputStream outputStream = new FileOutputStream(localFilePath);
// 下载文件
if (!ftpClient.retrieveFile(remoteFilePath, outputStream)) {
throw new IOException("下载文件失败:" + remoteFilePath);
}
// 关闭输出流
outputStream.close();
System.out.println("文件已成功下载到本地:" + localFilePath);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
// 断开连接
ftpClient.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
相关推荐
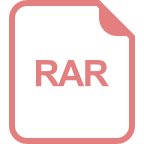
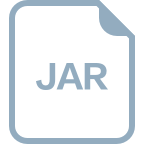
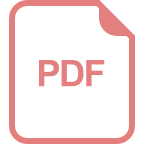














