java ftp 多线程下载文件
时间: 2024-02-01 07:10:36 浏览: 94
以下是Java实现FTP多线程下载文件的示例代码:
```java
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class FTPMultiThreadDownload {
private static final String FTP_SERVER = "ftp.example.com";
private static final int FTP_PORT = 21;
private static final String FTP_USERNAME = "username";
private static final String FTP_PASSWORD = "password";
private static final String REMOTE_FILE_PATH = "/path/to/remote/file";
private static final String LOCAL_FILE_PATH = "/path/to/local/file";
public static void main(String[] args) {
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(FTP_SERVER, FTP_PORT);
ftpClient.login(FTP_USERNAME, FTP_PASSWORD);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
int threadCount = 5; // 设置线程数量
ExecutorService executorService = Executors.newFixedThreadPool(threadCount);
long fileSize = ftpClient.listFiles(REMOTE_FILE_PATH)[0].getSize();
long partSize = fileSize / threadCount;
for (int i = 0; i < threadCount; i++) {
long startPos = i * partSize;
long endPos = (i + 1) * partSize - 1;
if (i == threadCount - 1) {
endPos = fileSize - 1;
}
executorService.execute(new DownloadThread(ftpClient, REMOTE_FILE_PATH, LOCAL_FILE_PATH, startPos, endPos));
}
executorService.shutdown();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
private static class DownloadThread implements Runnable {
private FTPClient ftpClient;
private String remoteFilePath;
private String localFilePath;
private long startPos;
private long endPos;
public DownloadThread(FTPClient ftpClient, String remoteFilePath, String localFilePath, long startPos, long endPos) {
this.ftpClient = ftpClient;
this.remoteFilePath = remoteFilePath;
this.localFilePath = localFilePath;
this.startPos = startPos;
this.endPos = endPos;
}
@Override
public void run() {
OutputStream outputStream = null;
try {
outputStream = new FileOutputStream(localFilePath, true);
ftpClient.setRestartOffset(startPos);
ftpClient.retrieveFile(remoteFilePath, outputStream);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (outputStream != null) {
outputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
请注意,上述代码使用了Apache Commons Net库来处理FTP操作。在代码中,我们首先建立与FTP服务器的连接,然后设置登录凭据和文件类型。接下来,我们确定要下载的文件的大小,并根据线程数量将文件分成多个部分。然后,我们使用线程池来创建并执行多个下载线程,每个线程负责下载文件的一个部分。每个线程都会将其下载的部分写入本地文件。最后,我们关闭连接和线程池。
阅读全文
相关推荐




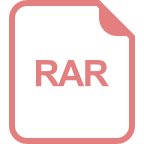







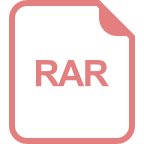

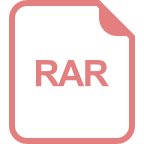



