如何在地图路径规划中应用最佳优先搜索算法来找到最优路径?请提供一个基于Java实现的示例。
时间: 2024-11-23 09:34:16 浏览: 13
在地图路径规划中,最佳优先搜索算法能有效地找到从起点到终点的最优路径,尤其是当搜索空间庞大时。算法的核心在于使用启发式评估函数来指导搜索方向,借助优先队列按照评估值的高低顺序选择节点进行扩展,以此来逼近目标节点。
参考资源链接:[最佳优先搜索算法详解:概念、Java实现及应用](https://wenku.csdn.net/doc/bx5t7d8qz8?spm=1055.2569.3001.10343)
要实现这一算法,可以参考《最佳优先搜索算法详解:概念、Java实现及应用》一书中的内容。在Java中实现最佳优先搜索,首先需要定义一个`Node`类,该类包含节点信息和启发式评估值,并重写`compareTo`方法以保证优先队列能够根据评估值正确排序节点。接下来,需要实现一个`BestFirstSearch`类,它将负责构建图的邻接矩阵表示,初始化搜索环境,并提供`findBestPath`方法来寻找最优路径。
以下是一个简化的Java示例代码,展示了如何使用最佳优先搜索算法进行地图路径规划:
```java
import java.util.*;
class Node implements Comparable<Node> {
int id; // 节点ID
double heuristicValue; // 启发式评估值
public Node(int id, double heuristicValue) {
this.id = id;
this.heuristicValue = heuristicValue;
}
@Override
public int compareTo(Node other) {
***pare(this.heuristicValue, other.heuristicValue);
}
}
public class BestFirstSearch {
private double[][] adjacencyMatrix; // 图的邻接矩阵
private PriorityQueue<Node> queue; // 优先队列
public BestFirstSearch(double[][] adjacencyMatrix) {
this.adjacencyMatrix = adjacencyMatrix;
this.queue = new PriorityQueue<>();
}
public List<Integer> findBestPath(int startId, int goalId) {
// 初始化起始节点并将其加入优先队列
Node startNode = new Node(startId, heuristic(startId, goalId));
queue.add(startNode);
// 已访问节点集合
Set<Integer> visited = new HashSet<>();
while (!queue.isEmpty()) {
Node currentNode = queue.poll();
int currentId = currentNode.id;
if (currentId == goalId) {
// 找到目标节点,返回路径
return constructPath(currentNode);
}
visited.add(currentId);
// 扩展当前节点,将相邻节点加入优先队列
for (int neighborId = 0; neighborId < adjacencyMatrix[currentId].length; neighborId++) {
if (adjacencyMatrix[currentId][neighborId] > 0 && !visited.contains(neighborId)) {
Node neighbor = new Node(neighborId, heuristic(neighborId, goalId));
queue.add(neighbor);
}
}
}
return null; // 未找到路径
}
// 启发式函数,计算节点到目标节点的估计成本
private double heuristic(int nodeId, int goalId) {
// 示例启发式函数,实际应用中应根据具体问题定义
return Math.abs(nodeId - goalId);
}
// 根据当前节点构造路径
private List<Integer> constructPath(Node currentNode) {
List<Integer> path = new ArrayList<>();
while (currentNode != null) {
path.add(0, currentNode.id); // 将当前节点添加到路径的开头
currentNode = currentNode.parent; // 获取父节点
}
return path;
}
public static void main(String[] args) {
// 示例邻接矩阵表示的图
double[][] adjacencyMatrix = {
// 初始化图的邻接矩阵...
};
BestFirstSearch search = new BestFirstSearch(adjacencyMatrix);
List<Integer> bestPath = search.findBestPath(startId, goalId);
// 输出最优路径
System.out.println(
参考资源链接:[最佳优先搜索算法详解:概念、Java实现及应用](https://wenku.csdn.net/doc/bx5t7d8qz8?spm=1055.2569.3001.10343)
阅读全文
相关推荐
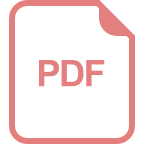
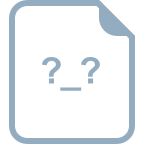
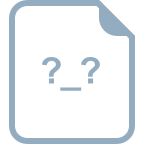




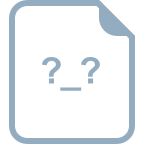
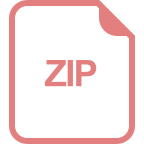
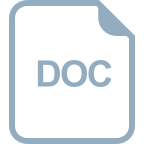
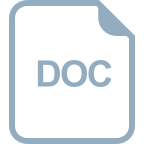
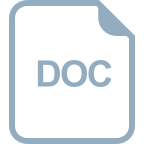
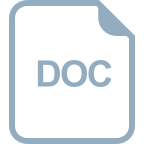
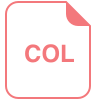
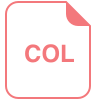



