图遍历与搜索策略在Java最短路径算法中的应用探究
发布时间: 2024-08-29 23:42:35 阅读量: 67 订阅数: 27 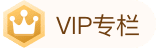
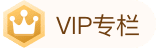
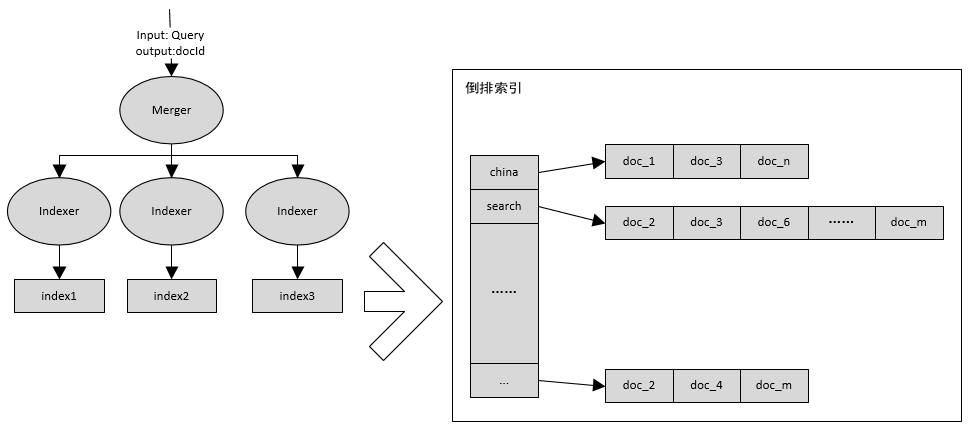
# 1. 图遍历与搜索策略基础
## 1.1 图遍历的基本概念
图遍历是图论中的一个基本问题,指的是从图中的一个顶点出发,访问图中所有顶点一次且仅一次。常见的图遍历算法包括深度优先搜索(DFS)和广度优先搜索(BFS)。DFS以深度优先的方式遍历,尝试沿着分支走尽可能深,直到到达尽头再回溯;而BFS则以广度优先的方式遍历,逐层向外扩展,访问所有邻接的节点后才移动到下一层。
## 1.2 搜索策略的重要性
在处理诸如网络路由、游戏树搜索等实际问题时,搜索策略起着至关重要的作用。选择合适的搜索策略可以显著影响算法的效率和结果的质量。例如,在路径规划问题中,一个合适的搜索策略能够帮助我们更快地找到最短路径。
## 1.3 搜索策略与算法实现
实现搜索策略时需要考虑算法的时间复杂度和空间复杂度。时间复杂度表征算法执行所需的时间量,空间复杂度则指算法执行所需存储空间的大小。对于图遍历,BFS的时间复杂度为O(V+E),空间复杂度为O(V),其中V表示顶点数,E表示边数。而DFS的时间复杂度同样为O(V+E),空间复杂度则为O(V),但需要额外的空间用于存储递归调用的栈。
通过选择合适的搜索策略,我们可以有效地解决图遍历中的各种问题,并为后续章节中将要介绍的最短路径算法奠定基础。在下一章中,我们将深入探讨Java中最短路径算法的理论基础,为实现具体的算法代码提供坚实的概念支撑。
# 2.2 搜索策略在最短路径中的作用
### 2.2.1 广度优先搜索(BFS)策略
广度优先搜索(Breadth-First Search,BFS)是图遍历算法中的一种,它从根节点开始,然后探索与根节点相邻的所有节点,再进一步探索与这些节点相邻的节点。BFS通常用于查找最短路径问题,尤其是在无权图中,因为它可以确保找到的路径是最短的。
BFS策略的关键在于使用一个队列来记录要访问的节点,它按照访问顺序的先进先出(FIFO)原则工作。BFS会按照从根节点出发的层次来访问所有节点,确保每层上的节点都访问过了才开始下一层的访问。
#### BFS算法步骤:
1. 将根节点加入到一个队列中。
2. 如果队列非空,则执行以下步骤:
a. 弹出队列的首节点。
b. 访问该节点,并将未访问过的相邻节点加入队列。
c. 重复步骤2直到队列为空。
BFS的Python代码实现:
```python
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(str(vertex) + " ", end="")
for neighbour in graph[vertex]:
if neighbour not in visited:
visited.add(neighbour)
queue.append(neighbour)
```
在这个代码示例中,`graph` 是一个字典,它存储了图的边,`start` 是起始节点。BFS从`start`开始,访问每个邻接节点,并将其加入到队列中。通过检查`visited`集合,我们确保每个节点只被访问一次,从而避免了重复。
### 2.2.2 深度优先搜索(DFS)策略
深度优先搜索(Depth-First Search,DFS)与BFS不同,它会尽可能深地遍历图的分支。当节点v的所有边都已被探寻过,搜索将回溯到发现节点v的那条边的起始节点。这个过程一直进行到已发现从源节点可达的所有节点为止。
DFS策略使用递归或栈来实现。使用递归实现时,如果当前节点没有子节点或所有子节点都被访问过,则回溯;使用栈实现时,如果当前节点没有子节点或所有子节点都被访问过,则栈顶元素出栈,实现回溯。
#### DFS算法步骤:
1. 创建一个空栈S和一个空集合visited。
2. 将起始节点压入栈S。
3. 如果栈S非空,则重复以下步骤:
a. 弹出栈顶元素,标记为当前节点。
b. 检查当前节点是否已访问过,若未访问过,将其加入到visited集合,并访问该节点。
c. 将当前节点的所有未访问的邻接节点压入栈S。
DFS的Python代码实现:
```python
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=" ")
for next_node in graph[start]:
if next_node not in visited:
dfs(graph, next_node, visited)
return visited
```
在这个代码示例中,`graph` 是一个字典,它存储了图的边,`start` 是起始节点。DFS从`start`开始,递归地访问每个未访问的邻接节点。注意,这种方法递归地进行,直到所有节点都被访问过为止。
### BFS与DFS在最短路径中的适用性
在无权图中,BFS和DFS都可以用来寻找最短路径。然而,由于BFS按照距离根节点的远近顺序访问节点,因此BFS可以用来找到从起点到所有其他节点的最短路径。DFS则不保证路径的最短性,因为它首先深入搜索,可能导致找到一个较长的路径。因此,在需要确定最短路径的场景中,BFS通常是更合适的选择。
# 3. Java实现的最短路径算法实践
在第二章中,我们深入了解了最短路径问题的理论基础和搜索策略。现在,让我们将理论应用于实践,探讨如何用Java实现这些算法。在本章中,我们将具体探讨Dijkstra算法、A*搜索算法和Floyd-Warshall算法,并展示如何用Java代码实现它们。
## 3.1 Dijkstra算法的Java实现
### 3.1.1 算法原理和步骤
Dijkstra算法是图论中最著名的算法之一,用于在加权图中找到两点之间的最短路径。算法原理基于贪心策略,始终保持一个起始点,计算最短距离,并逐步扩展到所有其他顶点。
Dijkstra算法的基本步骤如下:
1. 将所有顶点标记为未访问,并设置其距离为无穷大,除了起始顶点,其距离为0。
2. 创建一个优先队列(通常是最小堆),用于存储和选择最小距离的顶点。
3. 从优先队列中选择当前距离最小的未访问顶点。
4. 更新该顶点的邻接顶点的距离。
5. 标记当前顶点为已访问。
6. 重复步骤3到5,直到所有顶点都被访问。
### 3.1.2 Java代码实现和注释
```java
import java.util.*;
public class DijkstraAlgorithm {
// 定义图的边
static class Edge {
int src, dest, weight;
Edge() {
src = dest = weight = 0;
}
}
// 定义图的顶点
static class Graph {
int V, E;
List<Edge>[] array;
Graph(int V) {
this.V = V;
this.E = 0;
array = new LinkedList[V];
for (int i = 0; i < V; ++i)
array[i] = new LinkedList<>();
}
// 添加边到图中
void addEdge(int src, int dest, int weight) {
Edge edge = new Edge();
edge.src = src;
edge.dest = dest;
edge.weight = weight;
array[src].addFirst(edge); // 添加到邻接表
E++;
}
// 实现Dijkstra算法
void dijkstra(int src) {
PriorityQueue<Edge> pq = new PriorityQueue<>(V, (e1, e2) -> e1.weight - e2.weight);
boolean[] visited = new boolean[V];
// 将所有顶点的距离初始化为无穷大,并将源点距离设置为0
int[] dist = new int[V];
Arrays.fill(dist, Integer.MAX_VALUE);
dist[src] = 0;
pq.add(new Edge(src, src, 0));
while (!pq.isEmpty()) {
// 选择最小距离的顶点
Edge currentEdge = pq.poll();
int u = currentEdge.dest;
// 如果顶点已经被访问过了,则跳过
if (visited[u]) continue;
visited[u] = true;
// 遍历所有邻接顶点,更新距离
Iterator<Edge> i = array[u].listIterator();
while (i.hasNext()) {
Edge e = i.next();
int v = e.dest;
int weight = e.weight;
int nextDist = dist[u] + weight;
// 如果找到更短的路径,则更新距离并将其加入优先队列
if (!visited[v] && n
```
0
0
相关推荐
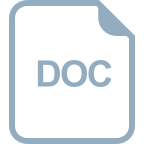
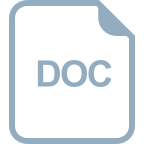
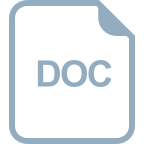
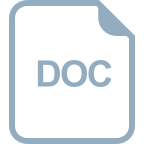
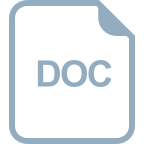
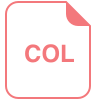
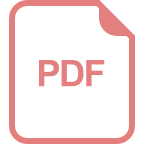
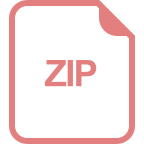
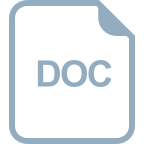