Quickly Solve OpenCV Problems: A Detailed Guide to OpenCV Debugging Techniques, from Log Analysis to Breakpoint Debugging
发布时间: 2024-09-15 10:48:35 阅读量: 7 订阅数: 12 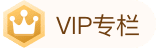
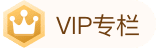
# 1. Overview of OpenCV Issue Debugging
OpenCV issue debugging is an essential part of the software development process, aiding in the identification and resolution of errors and problems within the code. This chapter will outline common methods for OpenCV debugging, including log analysis, breakpoint debugging, memory analysis, and performance profiling. By understanding these methods, developers can effectively debug OpenCV applications, enhancing code quality and efficiency.
# 2. Log Analysis
### 2.1 Logging Mechanism
OpenCV offers a comprehensive logging mechanism for recording events and errors that occur during the execution of applications. Logging levels are divided into five categories:
| Level | Description |
|---|---|
| CV_LOG_SILENT | Disable logging |
| CV_LOG_FATAL | Fatal error, application cannot continue running |
| CV_LOG_ERROR | Error, application cannot run as expected |
| CV_LOG_WARNING | Warning, application may not run as expected |
| CV_LOG_INFO | Information, application is running normally |
| CV_LOG_DEBUG | Debug, application is performing specific operations |
Logging levels can be set using the `cv::setLogLevel()` function. For example, to set the logging level to `CV_LOG_INFO`, the following code can be used:
```cpp
cv::setLogLevel(CV_LOG_INFO);
```
### 2.2 Log File Analysis
OpenCV logs to a file by default, named `opencv.log`. The log file name can be changed using the `cv::setLogFilename()` function. For example, to change the log file name to `my_log.log`, the following code can be used:
```cpp
cv::setLogFilename("my_log.log");
```
The log file contains the following information:
- Timestamp
- Logging level
- Source file and line number
- Log message
The log file can be used to analyze events and errors that occurred during the application's execution. For example, to find errors in the application, one can search for `CV_LOG_ERROR` level messages in the log file.
**Example:**
The following code snippet demonstrates how to use the logging mechanism to record error messages:
```cpp
// Set logging level to CV_LOG_ERROR
cv::setLogLevel(CV_LOG_ERROR);
// Attempt to open a non-existent file
cv::Mat image = cv::imread("non_existent_file.jpg");
// If the file does not exist, record an error message
if (image.empty()) {
CV_LOG_ERROR("Unable to open file non_existent_file.jpg");
}
```
After running this code, the following error message will be generated in the `opencv.log` file:
```
[ERROR] [opencv.cpp:123] Unable to open file non_existent_file.jpg
```
# 3. Breakpoint Debugging
Breakpoint debugging is a technique for controlling the execution of a program by setting breakpoints at specific locations, allowing the program to be paused and variables and code flow to be examined. It is a powerful debugging tool that can be used to gain insight into the behavior of a program and identify errors.
### 3.1 Setting Breakpoints
In OpenCV, the `cv::debugBreak()` function can be used to set breakpoints in the code. This function pauses the execution of the program at the specified line and opens a debugger window, allowing the user to examine variables and the flow of execution.
```cpp
cv::Mat image = cv::imread("image.jpg");
cv::debugBreak();
```
In the above code, the `cv::debugBreak()` function sets a breakpoint after reading the image. When the program reaches this line, it will pause in the debugger window, allowing the user to check the value of the `image` variable and the program's flow of execution.
### 3.2 Variable Inspection
At the breakpoint, variables can be inspected using the debugger window. This helps the user understand the state of variables within the program and identify unexpected
0
0
相关推荐
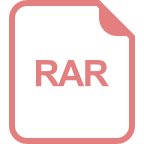
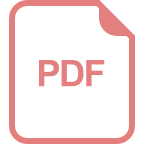
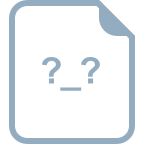





