【Full Analysis of Features from OpenCV Versions】: From 0.1 to 5.0, Witnessing the Evolutionary Journey of OpenCV
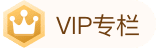
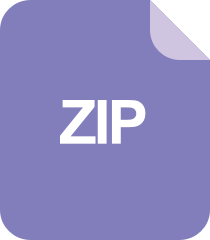
OpenCV 5.0 预览版压缩包几功能模块介绍;OpenCV 5.0 预览版压缩包几功能模块介绍;OpenCV 5.0 预览版
- Full Analysis of OpenCV Version Features: From 0.1 to 5.0, Witnessing the Evolutionary Journey of OpenCV
- 1. Overview of OpenCV
- 2. Evolution of OpenCV Versions
- 2.1 OpenCV 0.1-1.0: Foundation Construction and Image Processing
- 2.2 OpenCV 2.0-3.0: Breakthroughs in Machine Learning and Computer Vision
- 2.3 OpenCV 4.0-5.0: The Rise of Deep Learning and Mobile Development
- 3.1 Image Processing and Analysis
- 4. OpenCV Practical Applications
Full Analysis of OpenCV Version Features: From 0.1 to 5.0, Witnessing the Evolutionary Journey of OpenCV
1. Overview of OpenCV
OpenCV (Open Source Computer Vision Library) is an open-source computer vision library widely used in image processing, machine learning, and computer vision fields. It provides a series of powerful algorithms and functions for image processing, feature extraction, object detection, machine learning model training, and deployment.
Initially released by Intel Corporation in 1999, OpenCV has been continuously developed and updated since then. It initially focused on image processing but has gradually expanded its functionality to include machine learning, deep learning, and mobile development. OpenCV supports multiple platforms and can be used on Windows, Linux, macOS, and mobile devices.
2. Evolution of OpenCV Versions
2.1 OpenCV 0.1-1.0: Foundation Construction and Image Processing
The early versions of OpenCV (0.1-1.0) primarily focused on the foundational construction and functionality of image processing.
Code Example:
- import cv2
- # Read image
- image = cv2.imread('image.jpg')
- # Convert image to grayscale
- gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
- # Display image
- cv2.imshow('Original Image', image)
- cv2.imshow('Gray Image', gray_image)
- cv2.waitKey(0)
- cv2.destroyAllWindows()
Logical Analysis:
- The
cv2.imread()
function reads an image from a file path and stores it in theimage
variable. - The
cv2.cvtColor()
function converts the image from the BGR (Blue-Green-Red) color space to a grayscale image and stores it in thegray_image
variable. - The
cv2.imshow()
function displays the original and grayscale images. - The
cv2.waitKey(0)
function waits for the user to press any key to close the window. - The
cv2.destroyAllWindows()
function closes all open windows.
Parameter Explanation:
cv2.imread()
function:filename
: Image file path.
cv2.cvtColor()
function:image
: Input image.code
: Color space conversion code, in this case,cv2.COLOR_BGR2GRAY
.
cv2.imshow()
function:window_name
: Window name.image
: Image to display.
cv2.waitKey(0)
function:delay
: Milliseconds to wait for any key press, where0
means wait indefinitely.
cv2.destroyAllWindows()
function: No parameters.
2.2 OpenCV 2.0-3.0: Breakthroughs in Machine Learning and Computer Vision
The OpenCV 2.0-3.0 versions witnessed significant enhancements in machine learning and computer vision capabilities.
Code Example:
- import cv2
- # Use Haar cascade classifier for face detection
- face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
- # Read image
- image = cv2.imread('image.jpg')
- # Convert to grayscale
- gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
- # Face detection
- faces = face_cascade.detectMultiScale(gray_image, 1.1, 5)
- # Draw face bounding boxes on the image
- for (x, y, w, h) in faces:
- cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
- # Display image
- cv2.imshow('Detected Faces', image)
- cv2.waitKey(0)
- cv2.destroyAllWindows()
Logical Analysis:
- The
cv2.CascadeClassifier()
function loads a Haar cascade classifier for face detection. - The
cv2.detectMultiScale()
function detects faces in the grayscale image and returns the coordinates of the face bounding boxes. - The
cv2.rectangle()
function draws the face bounding boxes on the image. - The
cv2.imshow()
function displays the image with detected faces.
Parameter Explanation:
cv2.CascadeClassifier()
function:filename
: Path to the Haar cascade classifier file.
cv2.detectMultiScale()
function:image
: Input image.scaleFactor
: Scaling factor of the detection window size.minNeighbors
: Minimum number of faces detected in each detection window.
cv2.rectangle()
function:image
: Input image.pt1
: Coordinates of the top-left corner of the bounding box.pt2
: Coordinates of the bottom-right corner of the bounding box.color
: Color of the bounding box.thickness
: Thickness of the bounding box.
cv2.imshow()
function:window_name
: Window name.image
: Image to display.
2.3 OpenCV 4.0-5.0: The Rise of Deep Learning and Mobile Development
The OpenCV 4.0-5.0 versions introduced support for deep learning and mobile development, making it applicable in a broader range of scenarios.
Code Example:
- import cv2
- # Load a pre-trained deep learning model
- model = cv2.dnn.readNetFromCaffe('deploy.prototxt.txt', 'model.caffemodel')
- # Read image
- image = cv2.imread('image.jpg')
- # Preprocess the image
- blob = cv2.dnn.blobFromImage(image, 0.007843, (300, 300), 127.5)
- # Set input
- model.setInput(blob)
- # Forward propagation
- detections = model.forward()
- # Parse detection results
- for i in np.arange(0, detections.shape[2]):
- confidence = detections[0, 0, i, 2]
- if confidence > 0.2:
- x1 = int(detections[0, 0, i, 3] * image.shape[1])
- y1 = int(detections[0, 0, i, 4] * image.shape[0])
- x2 = int(detections[0, 0, i, 5] * image.shape[1])
- y2 = int(detections[0, 0, i, 6] * image.shape[0])
- cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
- # Display image
- cv2.imshow('Detected Objects', image)
- cv2.waitKey(0)
- cv2.destroyAllWindows()
Logical Analysis:
- The
cv2.dnn.readNetFromCaffe()
function loads a pre-trained deep learning model. - The
cv2.dnn.blobFromImage()
function preprocesses the image into a format suitable for the deep learning model. - The
model.setInput()
function sets the input data. - The
model.forward()
function performs forward propagation. - Parse the detection results, draw bounding boxes, and display the image.
Parameter Explanation:
cv2.dnn.readNetFromCaffe()
function:prototxt
: Path to the model deployment description file.caffemodel
: Path to the model weight file.
cv2.dnn.blobFromImage()
function:image
: Input image.scalefactor
: Image scaling factor.size
: Image size.mean
: Image mean.
model.setInput()
function:blob
: Input data.
model.forward()
function: No parameters.cv2.rectangle()
function:image
: Input image.pt1
: Coordinates of the top-left corner of the bounding box.pt2
: Coordinates of the bottom-right corner of the bounding box.color
: Color of the bounding box.thickness
: Thickness of the bounding box.
cv2.imshow()
function:window_name
: Window name.image
: Image to display.
3.1 Image Processing and Analysis
One of the core functions of OpenCV is image processing and analysis, which offers a range of powerful tools and algorithms that enable developers to perform various operations on images, including reading, transforming, displaying, enhancing, filtering, segmenting, and object detection.
3.1.1 Image Reading, Transformation, and Display
Image Reading
OpenCV provides various functions to read images, including:
- cv::imread(const std::string& filename, int flags = cv::IMREAD_COLOR);
Here, filename
is the path to the image file, and flags
specify the image reading mode (e.g., color, grayscale, transparency).
Image Transformation
OpenCV supports various image transformation operations, such as:
- cv::cvtColor(const cv::Mat& src, cv::Mat& dst, int code);
Here, src
is the source image, dst
is the target image, and code
specifies the transformation type (e.g., BGR to RGB, grayscale to color).
Image Display
OpenCV provides the imshow()
function to display images:
- cv::imshow(const std::string& winname, const cv::Mat& image);
Here, winname
is the window name, and image
is the image.
3.1.2 Image Enhancement and Filtering
Image Enhancement
OpenCV provides image enhancement algorithms, such as:
- cv::equalizeHist(const cv::Mat& src, cv::Mat& dst);
This function performs histogram equalization on the image, improving contrast.
Image Filtering
OpenCV provides a wide range of image filters, including:
- cv::GaussianBlur(const cv::Mat& src, cv::Mat& dst, cv::Size kernelSize, double sigmaX, double sigmaY);
This function applies Gaussian filtering to the image, blurring noise.
3.1.3 Image Segmentation and Object Detection
Image Segmentation
OpenCV provides image segmentation algorithms, such as:
- cv::kmeans(const cv::Mat& data, int K, cv::Mat& labels, cv::TermCriteria criteria, int attempts, cv::KMEANS_PP_CENTERS);
This function performs K-means clustering on the image, segmenting it into different regions.
Object Detection
OpenCV provides object detection algorithms, such as:
- cv::CascadeClassifier cascade;
- cascade.load("haarcascade_frontalface_default.xml");
This code loads a Haar cascade classifier for detecting faces in images.
4. OpenCV Practical Applications
4.1 Image Processing Practice
4.1.1 Face Recognition and Tracking
Face Recognition
Face recognition is a significant task in computer vision, capable of identifying and verifying individual identities. OpenCV provides a suite of face recognition algorithms, including:
- Face Detection: Haar cascade classifiers, deep learning models (e.g., MTCNN)
- Face Alignment: Algorithms for aligning eyes and nose
- Face Feature Extraction: Local Binary Patterns (LBP), Histogram of Oriented Gradients (HOG)
- Face Recognition: Principal Component Analysis (PCA), Linear Discriminant Analysis (LDA), Support Vector Machines (SVM)
Code Example:
- import cv2
- # Load face detection model
- face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
- # Load video stream
- cap = cv2.VideoCapture(0)
- while True:
- # Read frame
- ret, frame = cap.read()
- if not ret:
- break
- # Convert to grayscale
- gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
- # Face detection
- faces = face_cascade.detectMultiScale(gray, 1.3, 5)
- # Draw face rectangles
- for (x, y, w, h) in faces:
- cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
- # Display frame
- cv2.imshow('frame', frame)
- # Exit on 'q'
- if cv2.waitKey(1) & 0xFF == ord('q'):
- break
- # Release video stream
- cap.release()
- cv2.destroyAllWindows()
Logical Analysis:
- Load the face detection model: Use a Haar cascade classifier to detect faces.
- Load video stream: Read frames from a camera or video file.
- Convert to grayscale: Convert color frames to grayscale images to improve detection efficiency.
- Face detection: Use the face detection model to detect faces in the grayscale image.
- Draw face rectangles: Draw rectangles around detected faces.
- Display frame: Show frames containing detected faces.
- Exit on ‘q’: Exit the loop when the ‘q’ key is pressed.
Parameter Explanation:
1.3
: The scaling factor for the face detection model.5
: The minimum number of neighbors for the face detection model.
4.1.2 Image Stitching and Panorama Generation
Image Stitching
Image stitching is the process of combining multiple overlapping images into a single panoramic image. OpenCV provides a series of image stitching algorithms, including:
- Image Registration: Feature matching, image warping
- Image Blending: Feathering, multi-band blending
Panorama Generation
Panorama generation is the process of stitching multiple overlapping images into a 360-degree panoramic image. OpenCV provides a series of panorama generation algorithms, including:
- Spherical Projection: Projecting images onto a spherical surface
- Cylindrical Projection: Projecting images onto a cylindrical surface
Code Example:
- import cv2
- import numpy as np
- # Load images
- images = []
- for i in range(1, 5):
- img = cv2.imread(f'image{i}.jpg')
- images.append(img)
- # Image registration
- stitcher = cv2.Stitcher_create()
- status, pano = stitcher.stitch(images)
- # Display panorama
- if status == cv2.Stitcher_OK:
- cv2.imshow('pano', pano)
- cv2.waitKey()
- cv2.destroyAllWindows()
- else:
- print('Stitching failed')
Logical Analysis:
- Load images: Load the images to be stitched.
- Image registration: Use the Stitcher class to register the images.
- Image stitching: Use the Stitcher class to stitch the registered images into a panorama.
- Display panorama: Display the stitched panoramic image.
Parameter Explanation:
cv2.Stitcher_create()
: Create a Stitcher object.status
: Stitching status; ifcv2.Stitcher_OK
, stitching is successful.pano
: The stitched panoramic image.
5.1 OpenCV Environment Configuration and Optimization
Environment Configuration
Installing and configuring OpenCV is relatively straightforward, but some environment configurations are necessary to achieve optimal performance.
1. Dependency Library Installation
OpenCV relies on several external libraries, such as NumPy, SciPy, and Matplotlib. Ensure these libraries are installed before installing OpenCV.
2. OpenCV Installation
OpenCV can be installed in various ways, including:
- Using package managers (such as pip or conda)
- Compiling from source
- Using precompiled binaries
Using package managers is recommended as it is the simplest method.
3. Environment Variable Setup
After installing OpenCV, set environment variables to tell the system where to find the libraries and header files.
- Windows: Add the following environment variables in “System Properties”:
OPENCV_DIR
: Points to the OpenCV installation directoryPATH
: Add%OPENCV_DIR%\bin
- Linux/macOS: Add the following lines to the
.bashrc
or.zshrc
***export OPENCV_DIR=/path/to/opencv
export PATH=$PATH:$OPENCV_DIR/bin
Performance Optimization
Common methods for optimizing OpenCV performance include:
1. Using Optimized Compilers
Using an optimized compiler (such as Clang or GCC) can generate faster code.
2. Using Multithreading
OpenCV supports multithreading, which can improve the performance of image processing tasks.
3. Using GPU Acceleration
OpenCV can utilize GPU acceleration through CUDA or OpenCL, which can significantly increase processing speed.
4. Using Caching
Caching frequently accessed data can reduce I/O operations, thereby improving performance.
5. Using Appropriate Data Structures
Choosing appropriate data structures (such as matrices or arrays) can optimize code performance.
Code Example
The following code example demonstrates how to optimize OpenCV code to improve performance:
- import cv2
- # Using multithreading
- img = cv2.imread('image.jpg')
- gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
- edges = cv2.Canny(gray, 100, 200)
- # Using GPU acceleration
- gpu_img = cv2.cuda.GpuMat(img)
- gpu_gray = cv2.cuda.cvtColor(gpu_img, cv2.COLOR_BGR2GRAY)
- gpu_edges = cv2.cuda.Canny(gpu_gray, 100, 200)
- # Using caching
- cache = {}
- def get_image(path):
- if path not in cache:
- cache[path] = cv2.imread(path)
- return cache[path]
By using these optimization techniques, OpenCV code performance can be significantly improved.
6. Future Outlook and Trends for OpenCV
As an ever-evolving open-source library, the future development direction of OpenCV is of great interest. The following are some industry expert predictions on the future outlook and trends for OpenCV:
6.1 In-Depth Integration of Artificial Intelligence
The integration of artificial intelligence (AI) technology with OpenCV will continue to deepen. OpenCV will serve as the underlying framework for AI algorithms and models, providing robust image processing and analysis capabilities for computer vision and machine learning tasks.
6.2 Popularization of Cloud and Edge Computing
With the popularization of cloud and edge computing, OpenCV will be used to process large amounts of image and video data in distributed environments. This will enable real-time processing and analysis, thereby expanding the scope of OpenCV applications.
6.3 Optimization of Deep Learning Models
OpenCV will continue to optimize its support for deep learning models. This includes integrating new deep learning frameworks, providing optimizations for specific hardware platforms, and developing new algorithms and tools to improve the performance and efficiency of deep learning models.
6.4 Continuous Development for Mobile Platforms
The development of OpenCV for mobile platforms will continue to flourish. With the proliferation of smartphones and Internet of Things (IoT) devices, OpenCV will provide powerful image processing and computer vision capabilities for mobile applications.
6.5 Exploration of Emerging Technologies
OpenCV will also explore emerging technologies such as Augmented Reality (AR), Virtual Reality (VR), and Mixed Reality (MR). These technologies will provide OpenCV with new application areas, such as virtual try-ons, interactive games, and immersive experiences.
6.6 Community Collaboration and Contribution
The open-source nature of OpenCV will continue to promote community collaboration and contribution. Developers and researchers will continue to contribute to the development of OpenCV, adding new features, improving existing algorithms, and exploring new application areas.
相关推荐







