Comparison of OpenCV Versions: Functional Differences to Help You Choose the Optimal Version
发布时间: 2024-09-15 10:29:12 阅读量: 43 订阅数: 39 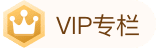
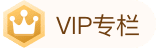
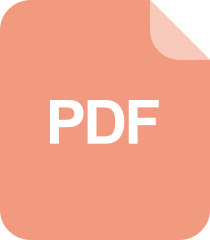
The severity of academic dishonesty: A comparison of faculty and student views
# 1. Introduction to OpenCV
OpenCV (Open Source Computer Vision Library) is an open-source computer vision library that offers a wide range of algorithms and functions for tasks such as image processing, video analysis, and machine learning. Initially developed by Intel, OpenCV has become one of the most popular libraries in the field of computer vision.
OpenCV provides a suite of features, including:
* Image processing: image enhancement, filtering, morphological operations, geometric transformations
* Video analysis: motion detection, object tracking, optical flow analysis
* Machine learning: classification, regression, clustering, dimensionality reduction
* Artificial intelligence: face recognition, object detection, scene understanding
# 2. Functional Differences Among Different Versions of OpenCV
OpenCV is a cross-platform computer vision library that has undergone several iterations since its inception, with each version bringing new features, improvements, and enhancements. Understanding the differences between various OpenCV versions is crucial for selecting the one that best suits the specific needs of your project.
### 2.1 OpenCV 1.x Series
The OpenCV 1.x series is the initial version of the library, released in 2006. It offers basic functions for computer vision and image processing, including:
- Image reading, writing, and conversion
- Image enhancement and filtering
- Morphological operations
- Feature detection and description
- Motion estimation
**Key Features and Characteristics:**
- **C++ Interface:** The OpenCV 1.x series uses a C++ interface, providing direct access to library functions and classes.
- **Cross-platform Support:** It supports multiple platforms, including Windows, Linux, and Mac OS X.
- **Community Support:** The OpenCV 1.x series has an active community that offers support and resources.
### 2.2 OpenCV 2.x Series
The OpenCV 2.x series was released in 2010 and introduced many new features and improvements, including:
- **Python Interface:** Alongside the C++ interface, the OpenCV 2.x series also introduced a Python interface, allowing developers to use the library more easily.
- **Modular Architecture:** The library is organized into modules, enabling developers to load only the functions they need, thus reducing the memory footprint of applications.
- **GPU Acceleration:** It supports GPU acceleration for certain operations, enhancing performance.
**New Features and Improvements:**
- **SURF and ORB Feature Detectors:** New feature detectors like SURF and ORB were introduced to improve the robustness of feature matching.
- **Optical Flow Estimation:** An optical flow estimation algorithm was added for tracking motion in video sequences.
- **Face Detection:** Face detection functionality was introduced, using Haar cascade classifiers.
### 2.3 OpenCV 3.x Series
The OpenCV 3.x series was released in 2015 and brought breakthrough updates and enhancements, including:
- **Deep Learning Support:** Support for deep learning was introduced, allowing developers to train and deploy deep learning models using OpenCV.
- **Mobile Device Support:** It supports mobile devices, providing functions optimized for mobile platforms.
- **OpenCL Acceleration:** It supports OpenCL-accelerated operations, further enhancing performance.
**Breakthrough Updates and Enhancements:**
- **TensorFlow and Caffe Integration:** Integration with deep learning frameworks such as TensorFlow and Caffe was added, making it easier for developers to integrate deep learning capabilities into their applications.
- **Mobile Device Optimization:** Optimizations for mobile devices were made, providing lightweight functions and algorithms to reduce memory usage and improve performance.
- **Machine Learning Algorithms:** New machine learning algorithms such as Support Vector Machines and Decision Trees were added.
### 2.4 OpenCV 4.x Series
The OpenCV 4.x series was released in 2018 and focused on core algorithm optimization and the introduction of new modules, including:
- **Core Algorithm Optimization:** The core algorithms were optimized for improved performance and accuracy.
- **New Modules:** New modules such as Dnn, Video, and Stitching were introduced to expand the library's capabilities.
- **Python 3 Support:** It supports Python 3, providing access to the latest version of the library.
**Core Algorithm Optimization and New Modules Introduction:**
- **DNN Module:** It provides support for deep neural networks, enabling developers to build and deploy deep learning models easily.
- **Video Module:** It offers video processing features such as video reading, writing, and analysis.
- **Stitching Module:** It provides image stitching capabilities for creating panoramic images.
**Table: Functional Differences Among Different Versions of OpenCV**
| Feature | OpenCV 1.x | OpenCV 2.x | OpenCV 3.x | OpenCV 4.x |
|---|---|---|---|---|
| C++ Interface | Yes | Yes | Yes | Yes |
| Python Interface | No | Yes | Yes | Yes |
| Modular Architecture | No | Yes | Yes | Yes |
| GPU Acceleration | No | Yes | Yes | Yes |
| Deep Learning Support | No | No | Yes | Yes |
| Mobile Device Support | No | No | Yes | Yes |
| OpenCL Acceleration | No | No | Yes | Yes |
| DNN Module | No | No | Yes | Yes |
| Video Module | No | No | Yes | Yes |
| Stitching Module | No | No | Yes | Yes |
**Code Examples:**
The following code examples demonstrate how to use face detection functionality in different versions of OpenCV:
```cpp
// OpenCV 1.x
#include <cv.h>
int main() {
IplImage* image = cvLoadImage("image.jpg");
CvHaarClassifierCascade* cascade = cvLoadHaarClassifierCascade("haarcascade_frontalface_default.xml");
CvSeq* faces = cvHaarDetectObjects(image, cascade, cvCreateMemStorage(0), 1.1, 3, 0, cvSize(30, 30));
for (int i = 0; i < faces->total; i++) {
CvRect* face = (CvRect*)cvGetSeqElem(faces, i);
cvRectangle(image, face->pt1, face->pt2, CV_RGB(255, 0, 0), 2);
}
cvShowImage("Faces", image);
cvWaitKey(0);
cvReleaseImage(&image);
cvReleaseHaarClassifierCascade(&cascade);
cvReleaseMemStorage(&storage);
return 0;
}
// OpenCV 2.x
#include <opencv2/opencv.hpp>
int main() {
Mat image = imread("image.jpg");
CascadeClassifier cascade("haarcascade_frontalface_default.xml");
std::vector<Rect> faces;
cascade.detectMultiScale(image, faces, 1.1, 3, 0, Size(30, 30));
for (size_t i = 0; i < faces.size(); i++) {
rectangle(image, faces[i], Scalar(255, 0, 0), 2);
}
imshow("Faces", image);
waitKey(0);
return 0;
}
// OpenCV 3.x
#include <opencv2/opencv.hpp>
int main() {
Mat image = imread("image.jpg");
String face_cascade_name = "haarcascade_frontalface_default.xml";
CascadeClassifier face_cascade;
if (!face_cascade.load(face_cascade_name)) {
std::cerr << "Error loading face cascade file" << std::endl;
return -1;
}
std::vector<Rect> faces;
face_cascade.detectMultiScale(image, faces, 1.1, 3, 0, Size(30, 30));
for (size_t i = 0; i < faces.size(); i++) {
rectangle(image, faces[i], Scalar(255, 0, 0), 2);
}
imshow("Faces", image);
waitKey(0);
return 0;
}
// OpenCV 4.x
#include <opencv2/opencv.hpp>
int main() {
Mat image = imread("image.jpg");
String face_cascade_name = "haarcascade_frontalface_default.xml";
Ptr<FaceDetectorYN> face_detector = createFaceDetectorYN(face_cascade_name);
std::vector<Rect> faces;
face_detector->detectMultiScale(image, faces, 1.1, 3, 0, Size(30, 30));
for (size_t i = 0; i < faces.size(); i++) {
rectangle(image, faces[i], Scalar(255, 0, 0), 2);
}
imshow("Faces", image);
waitKey(0);
return 0;
}
```
**Logical Analysis:**
The above code examples demonstrate how to use face detection functionality in different versions of OpenCV.
In OpenCV 1.x, the `cvLoadImage()` function is used to load the image, and then the `cvHaarDetectObjects()` function is used to detect faces.
In OpenCV 2.x, the `imread()` function is used to load the image, and then the `CascadeClassifier::detectMultiScale()` function is used to detect faces.
In OpenCV 3.x, the `imread()` function is used to load the image, and then the `CascadeClassifier::load()` function is used to load the face cascade classifier, and finally, the `CascadeClassifier::detectMultiScale()` function is used to detect faces.
In OpenCV 4.x, the `imread()` function is used to load the image, and then the `createFaceDetectorYN()` function is used to create a face detector.
# 3.1 Selection Based on Project Needs
When choosing the optimal version of OpenCV, project needs are a crucial consideration. Here are some aspects to consider:
#### 3.1.1 Consider Algorithm Requirements and Compatibility
Different versions of OpenCV offer different algorithms and features. It is essential to select a version that includes the necessary algorithms. For example, if you need to use deep learning algorithms, you must choose a version that supports these, such as OpenCV 4.x or higher.
In addition, consider compatibility with existing code and libraries. If you are using third-party libraries or code that depends on a specific version of OpenCV, you must select a compatible version.
### 3.2 Selection Based on Platform and Environment
The platform and environment can also affect the choice of OpenCV version. Here are some factors to consider:
#### 3.2.1 Consider the Operating System, Hardware Architecture, and Programming Language
OpenCV supports multiple operating systems, including Windows, Linux, and macOS. It is crucial to choose a version compatible with your operating system.
Additionally, consider the hardware architecture. OpenCV offers versions optimized for different hardware architectures, such as x86, ARM, and PowerPC. Choosing a version compatible with your hardware architecture can improve performance.
Finally, OpenCV can be used with various programming languages, including C++, Python, and Java. It is essential to choose a version that supports your preferred programming language.
### 3.3 Selection Based on Performance and Stability
Performance and stability are also important factors to consider when selecting a version of OpenCV. Here are some aspects to consider:
#### 3.3.1 Compare Performance and Stability on Specific Tasks for Different Versions
Different versions of OpenCV may have varying performance and stability on different tasks. It is advisable to compare the performance and stability of different versions on specific tasks before choosing a version.
For instance, you can use benchmarking tools to compare the performance of different versions on tasks such as image processing, computer vision, or machine learning. You can also look at online forums and community discussions to understand the stability of different versions.
# 4. OpenCV Version Upgrade Guide
### 4.1 Preparations Before Upgrading
#### 4.1.1 Back Up Code and Data
Before performing an OpenCV upgrade, it is crucial to back up all code and data. This will ensure that you can revert to the previous working state in case any issues arise during or after the upgrade.
#### 4.1.2 Confirm Compatibility
Before upgrading, you must ensure the compatibility of the new version of OpenCV with the existing code and system environment. This includes checking the following aspects:
- **Operating System Compatibility:** Ensure that the new version of OpenCV supports the current operating system.
- **Hardware Architecture Compatibility:** Confirm that the new version of OpenCV supports the current hardware architecture (e.g., x86, ARM).
- **Programming Language Compatibility:** Verify that the new version of OpenCV is compatible with the current programming language.
- **Third-party Library Compatibility:** Check if the new version of OpenCV is compatible with any dependent third-party libraries.
### 4.2 Upgrade Process
#### 4.2.1 Install the New Version
The steps to install the new version of OpenCV vary depending on the operating system and installation method. Here are some common methods:
- **Using Package Managers:** On Linux systems, you can use package managers (e.g., apt-get, yum) to install OpenCV.
- **Compiling from Source:** You can download the source code from the OpenCV official website and compile it.
- **Using Precompiled Binaries:** For some platforms, precompiled binaries can be downloaded from the OpenCV official website or third-party repositories.
#### 4.2.2 Adjust Code and Configuration
After installing the new version of OpenCV, you may need to adjust the code and configuration to be compatible with the new version. This may include:
- **Updating Header Files:** Replace old header files with the new version's header files.
- **Modifying Compiler Flags:** You may need to update compiler flags to use the new version of the OpenCV library.
- **Adjusting Configuration Settings:** Some configuration settings may need to be adjusted according to the new version of OpenCV.
### 4.3 Testing and Verification After Upgrading
#### 4.3.1 Run Test Cases
After upgrading, you should run test cases to verify the correct functionality of the new version of OpenCV. This will help identify any potential issues or incompatibilities.
#### 4.3.2 Check Results and Solve Problems
Carefully examine the results of the test cases and resolve any errors or issues that arise. This may involve debugging code, updating configurations, or seeking external support.
# 5. Future Development Outlook for OpenCV
### 5.1 Introduction of New Algorithms and Modules
As the fields of computer vision and machine learning continue to evolve, OpenCV will keep introducing new algorithms and modules to meet changing demands.
- **Computer Vision Algorithms:** OpenCV will integrate advanced computer vision algorithms, such as object detection, image segmentation, action recognition, and 3D reconstruction. These algorithms will enhance OpenCV's capabilities in image and video processing.
- **Machine Learning Modules:** OpenCV will incorporate machine learning modules, such as supervised learning, unsupervised learning, and deep learning. These modules will enable developers to build smarter, more powerful computer vision applications.
### 5.2 Performance Optimization and Acceleration
To meet the needs of real-time processing and high-performance computing, OpenCV will focus on optimizing its performance.
- **Multicore Processing:** OpenCV will leverage multicore processors, improving performance through parallel task processing.
- **GPU Acceleration:** OpenCV will support GPU acceleration, utilizing the powerful computing capabilities of graphics processing units to process image and video data.
- **Cloud Computing:** OpenCV will integrate with cloud platforms, providing scalable computing resources to handle large datasets and complex tasks.
### 5.3 Integration with Other Frameworks and Technologies
OpenCV will continue to integrate with other frameworks and technologies to offer more comprehensive solutions.
- **Deep Learning Frameworks:** OpenCV will integrate with deep learning frameworks such as TensorFlow and PyTorch, enabling developers to build end-to-end computer vision and machine learning solutions.
- **Cloud Platforms:** OpenCV will integrate with cloud platforms such as AWS and Azure, providing scalable computing resources and storage services.
- **Mobile Development Tools:** OpenCV will integrate with mobile development tools such as Android and iOS, allowing developers to build mobile computer vision applications.
0
0
相关推荐







